Declaration
property AlphaChannel: TIEBitmap;
Description
Returns the associated Alpha Channel of the image
Natively TIEBitmap handles the alpha channel as an encapsulated TIEBitmap object with
PixelFormat of ie8g. Pixels that are 0 (black) will be fully transparent, pixels of 255 are fully opaque, and values from 1 - 254 are partially transparent.
If an image doesn't have an alpha channel, accessing the AlphaChannel property will create it.
To determine whether an image has an alpha channel and/or contains transparent regions, check
HasAlphaChannel.
Note:
◼The
AlphaChannel must have a
PixelFormat of ie8g, and be the same width and height as the owner
TIEBitmap. If it is not, ImageEn will need to dynamically scale the channel, which will reduce performance. You can use
SyncAlphaChannel to automatically check and fix this if you modify the alpha channel
◼AlphaChannel is a standard
TIEBitmap, so you can use all
TIEBitmap methods, such as
Negative to invert the transparency
◼If you make changes to the
AlphaChannel of a bitmap displayed by
TImageEnView, you must call
Update to refresh the display
◼Remember that in the alpha channel, black is transparency, white is opacity (Imagine a night sky where the things in darkness/black are hidden, whereas those things in white/illumination are visible)
◼To use the "A" channel of a 32bit RGBA image, set
AlphaLocation to iaRGBA. Accessing the AlphaChannel will set
AlphaLocation to iaAlphaChannel
◼If
AutoOptimizeOnSave is enabled, the non-visible content of the image will be removed when saving
File Formats and Alpha
In order to maintain the alpha channel when saving, you will need to choose a format that supports it. The most widely used image format with true alpha support is PNG.
Alpha Channel is supported by:
◼BMP
◼CUR
◼ICO
◼IEN
◼JPEG 2000 with certain formats such as
ioJ2000_RGB
◼GIF, if
GIF_FlagTranspColor is enabled
◼PNG
◼PSD
◼SVG
◼TGA
◼TIFF (supported by some formats)
◼WIC formats, such as HDP, WDP, HEIF, etc.
◼WMF/EMF Metafiles (if
EMFBackgroundColor = clNone)
It is not supported by: AVI, Camera RAW, DCX, DICOM, JPEG, PCX
| Demos\ImageEditing\RGBChannels\RGBChannels.dpr |
| Demos\ImageEditing\CompleteEditor\PhotoEn.dpr |
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
| Demos\ImageEditing\AddBorder\AddBorder.dpr |
// Assign an alpha channel to an image using a source file
// For simple transparency, use a monochrome bitmap where the black pixels will become transparent
// Otherwise, a 256 color gray-scale image can provide a range of tranparency values (from white/fully opaque to black/fully transparent)
aBMP := TIEBitmap.create( 'D:\alpha.bmp' );
// Use our bitmap as the alpha channel
ImageEnView1.IEBitmap.AlphaChannel.Assign( aBMP );
// Ensure size of alpha channel matches size of image (and is ie8g)
ImageEnView1.IEBitmap.SyncAlphaChannel();
// Update the container
ImageEnView1.Update();
aBMP.Free();
Source Image
Alpha Image (Black will become transparent, gray will be 50% transparent and white will be fully opaque)
Result (on a white TImageEnView)
Example 2
// Feather the border of a JPEG image
iebmp.LoadFromFile( 'D:\image.jpg' );
iebmp.AlphaChannel; // Add alpha channel to image
iebmp.Resize(1, 1, 1, 1, clWhite, 0); // Add an alpha border
iebmp.FeatherAlphaEdges(10);
Example 3
// Create a shaped image, by drawing a letter to the canvas of the Alpha channel
ImageEnView1.IO.LoadFromFile( 'D:\Testing_Multimedia\Sub-100KB\Sunset 2.jpg' );
ImageEnView1.IEBitmap.AlphaChannel.Fill( clBlack );
ImageEnView1.IEBitmap.AlphaChannel.IECanvas.Font.Height := ImageEnView1.IEBitmap.Height;
ImageEnView1.IEBitmap.AlphaChannel.IECanvas.Font.Style := [fsBold];
ImageEnView1.IEBitmap.AlphaChannel.IECanvas.Font.Color := clWhite;
ImageEnView1.IEBitmap.AlphaChannel.IECanvas.DrawText( 'Q', Rect( 0, 0, ImageEnView1.IEBitmap.Width, ImageEnView1.IEBitmap.Height ), iejCenter );
ImageEnView1.Update();
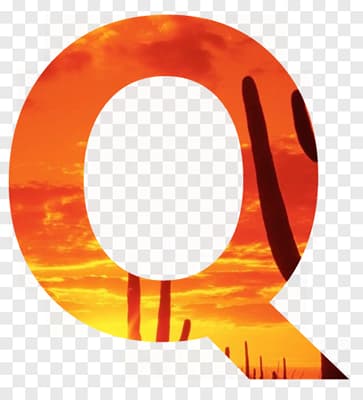
Example 4
// Create a shaped image, by drawing to the alpha channel
var aBmp := TIEBitmap.Create();
// Load our image content
aBmp.LoadFromFile( 'D:\fish.jpg' );
// Draw a shape to the alpha channel
aBmp.AlphaChannel.Fill( clBlack );
aBmp.AlphaChannel.FillWithShape( iesRadiance, -1, -1, clNone, 0, clWhite, False, True );
aBmp.SyncAlphaChannel(); // Fix the alpha channel
ImageEnView1.LayersAdd( aBmp ); // Do something with our image...
aBmp.Free();
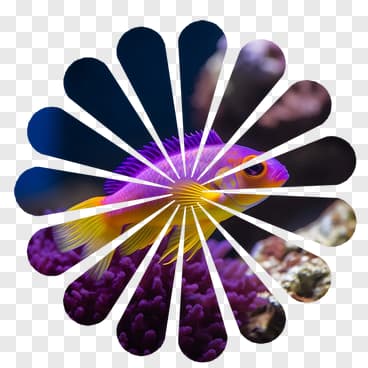
Example 5
// Show the percentage of pixels that are partially or fully transparent
// Same as TImageEnProc.CalcImageNumColors()
var
px: pbyte;
y, x, l: integer;
alphaCount, denom: Integer;
bmp: TIEBitmap;
begin
bmp := ImageEnView1.IEBitmap;
alphaCount := 0;
if bmp.HasAlphaChannel() then
begin
l := IEBitmapRowLen( bmp.AlphaChannel.Width, bmp.AlphaChannel.BitCount, 8);
for y := 0 to bmp.AlphaChannel.Height - 1 do
begin
px := bmp.AlphaChannel.Scanline[y];
for x := 0 to l - 1 do
begin
if px^ < 255 then
Inc( alphaCount );
inc(px);
end;
end;
end;
denom := bmp.Width * bmp.Height;
ShowMessage( format( 'Image has %d%% transparent pixels', [ Round( alphaCount / denom * 100 )]));
end;
See Also
◼Alpha
◼AlphaLocation
◼HasAlphaChannel
◼SyncAlphaChannel
◼DetachAlphaChannel
◼ReplaceAlphaChannel