Declaration
// Standard overload
procedure Crop(x1, y1, x2, y2 : Integer; FillColor: TColor = clWhite);
// TRect overload
procedure Crop(Rect : TRect; FillColor: TColor = clWhite);
// TRect with rotation overload. Angles are degrees clockwise
procedure Crop(Rect: TRect; Rotation: double; AntialiasMode: TIEAntialiasMode = ierFast);
// Perspective fix overload
procedure Crop(Quadrilater: array of TDPoint); overload;
Description
Replace the current image with that within the specified rectangle (i.e. keep only the specified region).
The Perspective fix overload allows you to adjust images that have perspective distortion. You need to specify the four points that will become the corners of the new image. The order is as follows:
◼Top-Left corner
◼Top-right corner
◼Bottom-right corner
◼Bottom-Left corner
Note:
◼Negative or out-of-range values will enlarge the image. FillColor will be used for the new areas. clNone can be specified for alpha fill
◼For other image editing functions, you can use
Proc
Rotate and Crop Explanation
The Rotate and Crop overload rotates the whole image and the crops to a region of interest by adjusting the crop region to account for the rotation.
Consider this image:
And these parameters:
const
Rotation_Angle = 10;
Crop_Left = 220;
Crop_Top = 70;
Crop_Right = 220 + 170;
Crop_Bottom = 70 + 160;
Cropping then rotating:
ImageEnView1.IO.LoadFromFile( 'D:\Tiger.jpg' );
ImageEnView1.Proc.Crop( Crop_Left, Crop_Top, Crop_Right, Crop_Bottom );
ImageEnView1.Proc.Rotate( Rotation_Angle, ierBicubic, clBlack );
Rotating then cropping:
ImageEnView1.IO.LoadFromFile( 'D:\Tiger.jpg' );
// Note the negative rotation value
ImageEnView1.Proc.Crop( Rect( Crop_Left, Crop_Top, Crop_Right, Crop_Bottom ), -Rotation_Angle, ierBicubic );
// Crop the image at position, Top-Left: (20, 20), Bottom-right: (100, 100). The resulting image will be 80 x 80 pixels
IEBmp.Crop( 20, 20, 100, 100 );
// Crop to the rect(100, 100, 300, 200) and rotate image 20 deg. Clock-wise
IEBmp.Crop( rect( 100, 100, 300, 200 ), 20, ierBicubic );
// Crops to the specified quadrilateral (4 points that will become the new corners) and applies forward perspective mapping. Useful to adjust perspective distortion
IEBmp.Crop([ DPoint(104, 85), // Top-Left corner
DPoint(1000, 150), // Top-right corner
DPoint(181, 500), // Bottom-right corner
DPoint(54, 400) // Bottom-Left corner
]);
// Fix a parallelogram
IEBmp.Crop([ DPoint(50, 0), DPoint(500, 0), DPoint(450, 400), DPoint(0, 400)]);
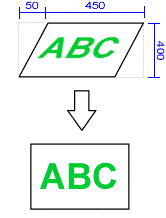
See Also
◼Resize
◼CropAlpha
◼Crop
◼Global Image Methods
◼Proc