Declaration
property EffectsChain: TIEImageEffectsList;
Description
Allows you to assign a batch of pending editing and color
operations to the bitmap, that are not immediately applied to the image (so they can be further tweaked).
While the EffectsChain is
enabled, any added effects will show in the image, but they can modified or removed. The changes are not locked into the image until
Apply is called.
Note:
◼You must enable the EffectsChain (by setting
Enabled) after loading your image
◼The effects chain is not stored with the image or transferred when assigning the bitmap to another one
| Demos\ImageEditing\EffectsChain\EffectsChain.dpr |
| Demos\InputOutput\BatchConvert\BatchConvert.dpr |
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
// Load an image
ImageEnView1.IO.LoadFromFile( 'D:\image.jpg' );
// Enable the effects chain
ImageEnView1.IEBitmap.EffectsChain.Enabled := True;
// Prompt the user to specify a color adjustment
ImageEnView1.IEBitmap.EffectsChain.Add( ppeColorAdjustments );
// Add a horizontal flip
ImageEnView1.IEBitmap.EffectsChain.Add( peRotate ); // Rotation and flipping type
ImageEnView1.IEBitmap.EffectsChain.CurrentItem.Flip_Horz := True;
ImageEnView1.Update(); // Must call update after manually setting properties
// Discard all added effects (revert image to original)
ImageEnView1.IEBitmap.EffectsChain.Clear();
// OR
// Permanently apply specified effects to the image (will clear the effects from the chain)
ImageEnView1.IEBitmap.EffectsChain.Apply();
Example 2
// Apply chain of editing operations to an image
var
op: TIEImageEffect;
begin
ImageEnView1.IO.LoadFromFile( 'D:\image.jpg' );
ImageEnView1.IEBitmap.EffectsChain.Enabled := True;
op := TIEImageEffect.Create();
try
// Reduce size by half
op.Operation := peResize;
op.Resize_Width := 50;
op.Resize_Height := 50;
op.Resize_ByPercent := True;
op.Resize_QualityFilter := rfLanczos3;
ImageEnView1.IEBitmap.EffectsChain.Add( op );
// Enhance contrast
op.Operation := peContrast;
op.Contrast_Contrast := 20;
ImageEnView1.IEBitmap.EffectsChain.Add( op );
// Rotate 45 deg. clockwise
op.Operation := peRotate;
op.Rotate_Angle := -45;
op.Rotate_BackgroundColor := clBlack;
op.Rotate_Antialias := ierFast;
ImageEnView1.IEBitmap.EffectsChain.Add( op );
// Apply effects
ImageEnView1.IEBitmap.EffectsChain.Apply();
ImageEnView1.IO.SaveToFile( 'D:\image-Edit.jpg' );
finally
op.Free;
end;
end;
Other Examples
// Prompt the user to specify a color adjustment, e.g. hue, contrast, automatic-enhancement, etc.
ImageEnView1.IEBitmap.EffectsChain.Insert( 0, ppeColorAdjustments );
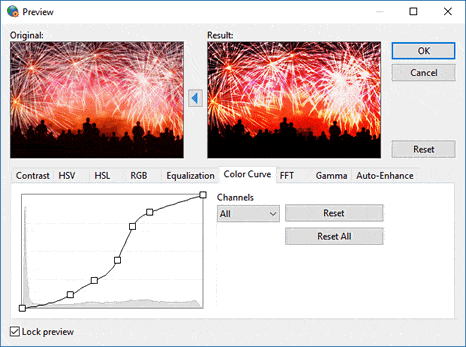
// Prompt the user to specify an editing effect, e.g. rotation, resizing or cropping
ImageEnView1.IEBitmap.EffectsChain.Insert( 0, ppeEditingFunctions );
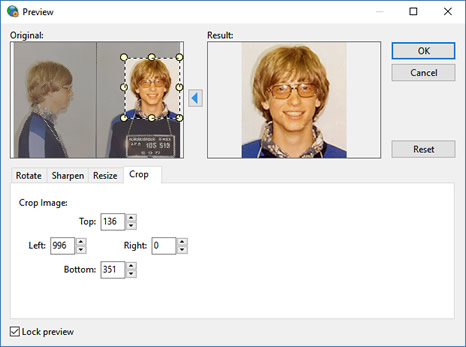
// Prompt the user to specify an image effect, e.g. Lens or Wave effect
ImageEnView1.IEBitmap.EffectsChain.Insert( 0, ppeSpecialEffects );
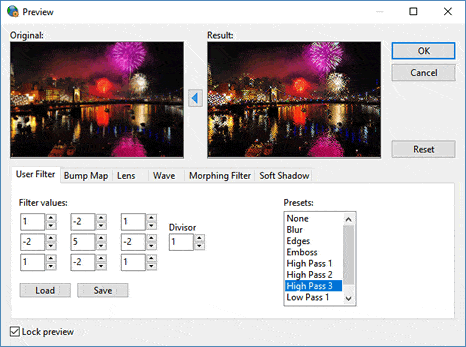
// Prompt the user to specify image rotation
ImageEnView1.IEBitmap.EffectsChain.Insert( 0, [peRotate] );
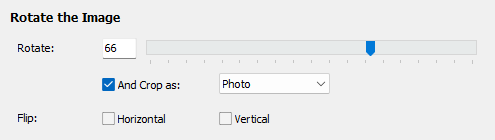
// Add a rotation effect to the image chain
ImageEnView1.IEBitmap.EffectsChain.Enabled := True;
ImageEnView1.IEBitmap.EffectsChain.Add( peRotate );
ImageEnView1.IEBitmap.EffectsChain.CurrentItem.Rotate_Angle := 90;
ImageEnView1.Update(); // Must call update after manually setting properties
// Progressively increase the image rotation by 1 deg. using an image chain (so there is no quality lost)
ImageEnView1.IEBitmap.EffectsChain.Enabled := True;
idx := ImageEnView1.IEBitmap.EffectsChain.EffectToIndex( peRotate );
if idx = -1 then
idx := ImageEnView1.IEBitmap.EffectsChain.Add( peRotate );
ImageEnView1.IEBitmap.EffectsChain.Items[idx].Rotate_Angle := ImageEnView1.IEBitmap.EffectsChain.Items[idx].Rotate_Angle + 1;
ImageEnView1.IEBitmap.EffectsChain.Items[idx].Rotate_Antialias := ierBicubic;
ImageEnView1.Update(); // Must call update after manually setting properties
// Add an operation to resize image to 1000x1000 (maintaining aspect ratio)
ImageEnView1.IEBitmap.EffectsChain.Add( peResize );
ImageEnView1.IEBitmap.EffectsChain.CurrentItem.Resize_Width := 1000;
ImageEnView1.IEBitmap.EffectsChain.CurrentItem.Resize_Height := 1000;
ImageEnView1.IEBitmap.EffectsChain.CurrentItem.Resize_ByPercent := False;
ImageEnView1.IEBitmap.EffectsChain.CurrentItem.Resize_QualityFilter := rfLanczos3;
ImageEnView1.Update(); // Must call update after manually setting properties
// If Contrast effect has been added, then modify it. Otherwise add it
idx := ImageEnView1.IEBitmap.EffectsChain.EffectToIndex( peContrast );
if idx = -1 then
idx := ImageEnView1.IEBitmap.EffectsChain.Add( peContrast );
ImageEnView1.IEBitmap.EffectsChain.Items[idx].Contrast_Contrast := trkContrast.Position;
ImageEnView1.Update(); // Must call update after manually setting properties
// If HSV effect has been added, then modify it. Otherwise add it
idx := ImageEnView1.IEBitmap.EffectsChain.EffectToIndex( peHSV );
if idx = -1 then
idx := ImageEnView1.IEBitmap.EffectsChain.Add( peHSV );
ImageEnView1.IEBitmap.EffectsChain.Items[idx].HSV_H := trkHsvH.Position;
ImageEnView1.Update(); // Must call update after manually setting properties
// Prompt user to edit the selected effect
ImageEnView1.IEBitmap.EffectsChain.Edit( cbxEffects.ItemIndex );
// Disable all the effects (but don't delete them)
for i := 0 to ImageEnView1.IEBitmap.EffectsChain.Count - 1 do
ImageEnView1.IEBitmap.EffectsChain.Items[i].Enabled := False;
ImageEnView1.Update(); // Must call update after manually setting properties
// Load effects chain from file and apply the effects to a list of images
bmp := TIEBitmap.Create();
for i := 0 to ssFiles.Count - 1 do
begin
bmp.LoadFromFile( ssFiles[i] );
bmp.EffectsChain.Enabled := True;
bmp.EffectsChain.LoadFromFile( 'D:\Effects.ieeff' );
bmp.EffectsChain.Apply();
bmp.SaveToFile( ChangeFileExt( ssFiles[i], '_convert.jpg' );
end;
bmp.Free;
// Save effects chain to file
if SaveDialog1.Execute() then
ImageEnView1.IEBitmap.EffectsChain.SaveToFile( SaveDialog1.FileName );
// Apply effects in a chain file to current image
if OpenDialog1.Execute() then
begin
ImageEnView1.IEBitmap.EffectsChain.Enabled := True;
ImageEnView1.IEBitmap.EffectsChain.LoadFromFile( OpenDialog1.FileName );
ImageEnView1.IEBitmap.EffectsChain.Apply();
end;
// Display the active list of effects assigned to the image
cbxEffects.Clear();
for i := 0 to ImageEnView1.IEBitmap.EffectsChain.Count - 1 do
begin
cbxEffects.Items.Add( ImageEnView1.IEBitmap.EffectsChain.Items[i].Description() );
cbxEffects.Checked[i] := ImageEnView1.IEBitmap.EffectsChain.Items[i].Enabled;
end;
// Disable button if there are no effects assigned to the image
btnClear.Enabled := ImageEnView1.IEBitmap.EffectsChain.Count > 0;
// Disable button if there is already a soft shadow applied
btnAddSoftShadow.Enabled := ImageEnView1.IEBitmap.EffectsChain.EffectToIndex( peSoftShadow ) = -1;
// Disable button if there is already a horizontal applied
btnAddHorzFlip.Enabled := ImageEnView1.IEBitmap.EffectsChain.EffectToIndex( peRotate, IPP_FLIP_HORZ, True ) = -1;
// Add an operation to add a 5 pixel black border to the image
ImageEnView1.IEBitmap.EffectsChain.Add( peCrop ); // We will do a negative crop
ImageEnView1.IEBitmap.EffectsChain.CurrentItem.Crop_Left := -5;
ImageEnView1.IEBitmap.EffectsChain.CurrentItem.Crop_Top := -5;
ImageEnView1.IEBitmap.EffectsChain.CurrentItem.Crop_Right := -5;
ImageEnView1.IEBitmap.EffectsChain.CurrentItem.Crop_Bottom := -5;
ImageEnView1.IEBitmap.EffectsChain.CurrentItem.Crop_BackgroundColor := clBlack;
ImageEnView1.Update(); // Must call update after manually setting properties