Declaration
TIEMouseInteractLayerItems = (mlMoveLayers, mlResizeLayers, mlRotateLayers, mlCreateImageLayers, mlCreateShapeLayers, mlCreateLineLayers, mlCreatePolylineLayers, mlCreateAngleLayers, mlCreateTextLayers, mlClickCreateLineLayers, mlClickCreatePolylineLayers, mlDrawCreatePolylineLayers, mlClickCreateAngleLayers, mlEditLayerPoints);
TIEMouseInteractLayers = set of TIEMouseInteractLayerItems;
Description
Actions that occur when the user moves or clicks the mouse, which are related to creating and editing layers.
Also see
TIEMouseInteract, which are general display and editing interactions.
Value | Description |
mlClickCreateAngleLayers | Click three points to create a TIEAngleLayer (Notes 1,2). Click Esc to cancel insertion |
mlClickCreateLineLayers | Click start and end points to create a TIELineLayer (Notes 1,2). Click Esc to cancel insertion |
mlClickCreatePolylineLayers | Click multiple points to create a TIEPolylineLayer (Notes 1,2). Click Enter, Double-click, or click near the start point to complete the polyline. Use LayersAutoClosePolylines to determine whether the polyline is closed (i.e. becomes a polygon). Click Esc to cancel insertion, or DEL to delete the last added point |
mlDrawCreatePolylineLayers | Click and drag to freehand draw a TIEPolylineLayer (Notes 1,2) |
mlCreateImageLayers | Click and drag the mouse to create a TIEImageLayer (Notes 1,3) |
mlCreateShapeLayers | Click and drag the mouse to create a TIEShapeLayer (Notes 1,3) |
mlCreateLineLayers | Click and drag the mouse to create a TIELineLayer (Notes 1,3) |
mlCreateAngleLayers | Click and drag the mouse to create a TIEAngleLayer (Notes 1,3) |
mlCreatePolylineLayers | Click and drag the mouse to create a TIEPolylineLayer (Notes 1,3). This creates area of the layer, you will need to add points |
mlCreateTextLayers | Click and drag the mouse to create a TIETextLayer (Notes 1,3) |
mlEditLayerPoints | Click and drag points of a TIELineLayer or TIEPolylineLayer to move them. Click on the polyline to add a point, Alt key to convert a point to a curve, Ctrl+click or delete key to remove a point. Hold Alt key, then click on a line layer and drag to convert to a curve (Note 2,4) |
mlMoveLayers | User can move layers |
mlResizeLayers | User can resize layers using grips. Also see: LayersFixSizes |
mlRotateLayers | User can rotate layers by dragging the rotation grip (if loShowRotationGrip is enabled), otherwise clicking and dragging anywhere outside the layer (Notes 2,5). Also see: LayersFixRotations |
Note:
1. If you are unable to create layers on the background, ensure that ImageEnView1.Layers[0].
Selectable = False.
2. Hold Shift key to lock line angles to
LayersRotateStep
3. If
loStampMode is specified for
LayerOptions, a single click will add the layer
4. Hold Alt key to convert point to a curve. Alt+Shift will give an exact half circle. Use
LayersCurvePoints to specify how many points are used for a curve
5. Use
mlRotateLayers in layer applications, and
miRotateTool in other (single layer) applications
Usage Notes
You can only select one of the following:
mlCreateImageLayers, mlCreateShapeLayers, mlCreateLineLayers, mlCreatePolylineLayers, mlCreateAngleLayers, mlCreateTextLayers,
mlClickCreateLineLayers, mlClickCreatePolylineLayers, mlDrawCreatePolylineLayers, mlClickCreateAngleLayers
The following are often used in combinations with others:
miZoom, miSmoothZoom, miDblClickZoom, miMovingScroll, mlEditLayerPoints,
mlMoveLayers, mlResizeLayers, mlRotateLayers (can be used with any of the
miSelect* methods, except miSelectZoom). When miSelect and mlMoveLayer are specified together, then movement is possible only by clicking and dragging the edge of the current layer.
To use mlRotateLayers in combination with layer creation interactions (mlCreate*Layers),
loShowRotationGrip must be included for
LayerOptions
if mlEditLayerPoints and mlResizeLayers are used in combination, point layers will only be resizable by dragging their points
Common Usages
Layer Creation (create, move and resize layers):
ImageEnView1.MouseInteractLayers := [ mlCreate*Layers, mlMoveLayers, mlResizeLayers ]; // Note: Not mlRotateLayers
Layer Editing (move, resize and rotate layers):
ImageEnView1.MouseInteractLayers := [ mlMoveLayers, mlResizeLayers, mlRotateLayers ];
Polyline Layer Creation (create and edit polyline layers):
ImageEnView1.MouseInteractLayers := [ mlClickCreatePolylineLayers, mlEditLayerPoints ];
Line Layer Creation (create and edit line layers):
ImageEnView1.MouseInteractLayers := [ mlClickCreateLineLayers, mlEditLayerPoints ];
Angle Layer Creation (create and edit angle layers):
ImageEnView1.MouseInteractLayers := [ mlClickCreateAngleLayers, mlEditLayerPoints ];
Line, Angle and Polyline Layer Editing (move, point editing, resizing and rotation):
ImageEnView1.MouseInteractLayers := [ mlMoveLayers, mlEditLayerPoints, mlRotateLayers, mlResizeLayers ];
Selections in a multi-layer app
ImageEnView1.MouseInteractGeneral := [ miSelect, mlMoveLayers ];
| Demos\Other\MouseInteract\MouseInteract.dpr |
| Demos\LayerEditing\RotateLayers\RotateLayers.dpr |
| Demos\LayerEditing\Layers_AllTypes\Layers.dpr |
| Demos\LayerEditing\Layers_Lines\Layers.dpr |
// Allow users to create image layers. Prompt for an image file after selection
ImageEnView1.LayerOptions := ImageEnView1.LayerOptions + [ loAutoPromptForImage ];
ImageEnView1.MouseInteractLayers := [ mlCreateImageLayers ];
// Allow user to move and resize layers (allow multiple layer selection and ensure masks are moved with layers)
ImageEnView1.LayerOptions := ImageEnView1.LayerOptions + [ loAllowMultiSelect, loAutoSelectMask ];
ImageEnView1.MouseInteractLayers := [ mlMoveLayers, mlResizeLayers ];
A selected text layer with resize grips:
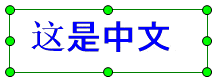
Four selected layers with resize grips:
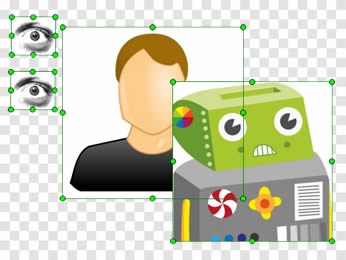
// Allow user to rotate layers (allow multiple layer selection and ensure masks are moved with layers)
ImageEnView1.LayerOptions := ImageEnView1.LayerOptions + [ loAllowMultiSelect, loAutoSelectMask ];
ImageEnView1.MouseInteractLayers := [ mlRotateLayers ];
// Allow user to make selections when there are multiple layers (current layer will be highlighted. Selecting a new layer will highlight and allow selection. Layer movement is possible by dragging the edge of the layer)
ImageEnView1.MouseInteractGeneral := [ miSelect, mlMoveLayers ];
// Allow user to make selections when there are multiple layers, including resizing and rotating layers. To move layers, you must drag and click the layer edge
ImageEnView1.MouseInteractGeneral := [ miSelect, mlMoveLayers, mlResizeLayers, mlRotateLayers ];
// Allow users to create text layers. Start in text editing mode (user can immediately begin typing text)
ImageEnView1.LayerOptions := ImageEnView1.LayerOptions + [ loAutoTextEditing ];
ImageEnView1.MouseInteractLayers := [ mlCreateTextLayers ];
// Add a star every time the layer clicks on the image (use OnNewLayer event to customize size)
ImageEnView1.LayerOptions := ImageEnView1.LayerOptions + [ loStampMode ];
IEGlobalSettings().DefaultLayerShape := iesStar5;
ImageEnView1.MouseInteractLayers := mlCreateShapeLayers;
// Allow user to draw a polygon
ImageEnView1.LayersAutoClosePolylines := iecmAlways;
ImageEnView1.MouseInteractLayers := [ mlClickCreatePolylineLayers ];
// Allow users to create and edit a polyline
ImageEnView1.LayersAutoClosePolylines := iecmManual;
ImageEnView1.MouseInteractLayers := [ mlClickCreatePolylineLayers, mlEditLayerPoints ];
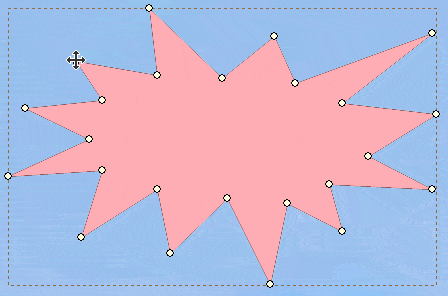
See Also
◼MouseInteractLayers
◼MouseInteractGeneral
◼SelectionOptions
◼LayerDefaults
◼LayersAutoClosePolylines