ImageEn, unit iexHelperFunctions |
|
IEDrawShape
Declaration
procedure IEDrawShape(Canvas: TCanvas;
Shape: TIEShape;
Left, Top, Width, Height: Integer;
MaintainAspect: TIEBooleanEx = iebDefault); overload;
procedure IEDrawShape(Canvas: TCanvas;
Shape: TIEShape;
Left, Top, Width, Height: Integer;
MaintainAspect: TIEBooleanEx;
BorderColor: TColor; BorderWidth: Integer;
FillColor: TColor;
Angle: Integer = 0;
ShapeModifier: Integer = 0); overload;
procedure IEDrawShape(Canvas: TIECanvas;
Shape: TIEShape;
Left, Top, Width, Height: Integer; MaintainAspect: TIEBooleanEx;
BorderColor: TColor; BorderWidth: Integer;
FillColor: TColor; FillColor2: TColor = clNone; FillGradient: TIEGDIPlusGradient = gpgVertical;
AntiAlias: Boolean = True;
Angle: Integer = 0;
ShapeModifier: Integer = 0;
BorderTransparency: Integer = 255; FillTransparency: Integer = 255); overload;
Description
Draw a
TIEShape onto a canvas at the specified size and position.
If MaintainAspect is
iebDefault, then shapes are output at the aspect ratio they were designed (if
iebTrue then a 1:1 aspect ratio is enforced even if it was not designed with one).
For the first overload, the Canvas Brush is used for color and fill.
For the second, the colors you specify will override the canvas brush.
Angle is the amount of rotation to apply (degrees counter-clockwise).
ShapeModifier has the following effect:
Shape | Effect | Range | Default |
iesCustomShape | Number of sides of the shape | 3 - 50 | 10 |
iesCustomStar | Number of points of the star | 3 - 50 | 10 |
iesCustomExplosion | Number of points of the explosion | 3 - 50 | 12 |
iesEllipseSegment | Width of the ellipse segment (in degrees) | 1 - 90 | 45 |
iesCustomCog | Number of teeth of the cog | 3 - 72 | 12 |
iesCustomAsterisk | Number of points of the asterisk | 3 - 72 | 12 |
iesCustomFlower | Number of petals of the flower | 3 - 72 | 12 |
iesCustomRadiance | Number of rays | 3 - 72 | 12 |
iesCustomRadiance2 | Number of rays | 3 - 72 | 12 |
Note:
◼You must add the iexHelperFunctions unit to your uses clause
◼The TIECanvas overload is the same as calling
AdvancedDrawShape
// Draw a pink balloon
ImageEnView1.IEBitmap.Allocate( 600, 800 );
ImageEnView1.IEBitmap.Fill( clWhite );
IEDrawShape( ImageEnView1.IEBitmap.IECanvas, iesBalloon, 0, 0, ImageEnView1.IEBitmap.Width, ImageEnView1.IEBitmap.Height, iebDefault, clBlack, 2, $00921CF0 );
ImageEnView1.Update();
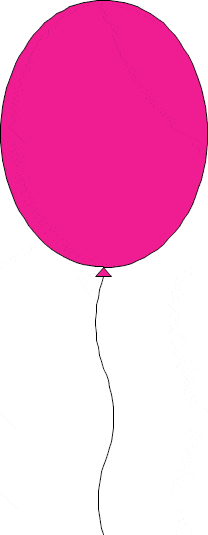
// Draw a explosion at the centre of the current image
IEDrawShape( ImageEnView1.IEBitmap.Canvas, iesExplosion, Width div 2 - 100, Height div 2 - 100, 200, 200 );
ImageEnView1.Update;
// Draw a shape to the current the image
IEDrawShape( ImageEnView1.IEBitmap.IECanvas, // Dest canvas
iesHeart, // Shape
60, 60, 180, 180, // Position
iebDefault, // MaintainAspect
clRed, 10, // BorderColor/BorderWidth
clNone, clNone, gpgVertical ); // FillColor/FillColor2/FillGradient: TIEGDIPlusGradient
ImageEnView1.Update();
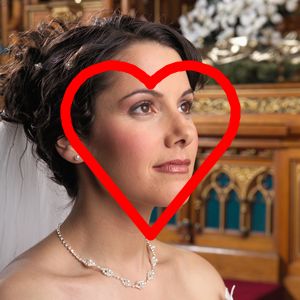
// Add all shapes to a TImageEnMView
ImageEnMView1.LockUpdate();
ImageEnMView1.Clear;
for shp := Low(TIEShape) to High(TIEShape) do
begin
bmp := TBitmap.Create;
bmp.PixelFormat := pf24bit;
bmp.Width := Thumb_Size;
bmp.Height := Thumb_Size;
IEDrawShape( bmp.Canvas, shp, 0, 0, bmp.Width, bmp.Height, iebDefault, clNone, 0, clRed );
idx := ImageEnMView1.AppendImage( bmp );
ImageEnMView1.ImageBottomText[idx] := IEShapeToStr( shp );
bmp.free;
end;
ImageEnMView1.UnlockUpdate();
// Save all shapes as gif files
for shp := Low(TIEShape) to High(TIEShape) do
begin
bmp := TIEBitmap.Create;
bmp.Allocate( Thumb_Size, Thumb_Size );
bmp.Fill(clWhite );
IEDrawShape( bmp.IECanvas, shp, 0, 0, bmp.Width, bmp.Height, iebDefault, clNone, 0, $00FF8000 );
bmp.SaveToFile( 'D:\' + IEShapeToStr( shp ) +'.gif');
bmp.Free();
end;
See Also
◼IEDrawShapeToComboListBoxItem
◼FillWithShape
◼AdvancedDrawShape