ImageEn, unit imageenproc |
|
TImageEnProc.ConvertToBWThreshold
Declaration
function ConvertToBWThreshold(Threshold: Integer = -1): Integer;
Description
Convert a true color image (24 bit) to black & white (1 bit) using a thresholding algorithm.
The image is first converted to gray levels, then all levels less than Threshold are set to black, while the remainder are set to white.
Threshold is an intensity value (0..255).
If Threshold is -1, the threshold value used will be the average level of the original image.
If Threshold is -2, a Maximum Entropy Algorithm is used.
Result is the threshold that was applied (e.g. if threshold was passed as -1, the result will be the average threshold level). If the image cannot be processed (e.g. it is already black & white), the result will be -1
Note: Any selection is ignored. The whole image is converted to the specified palette
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
Black and White Conversion Comparison
ImageEnView1.IO.LoadFromFile( 'D:\TestImage.jpg' );
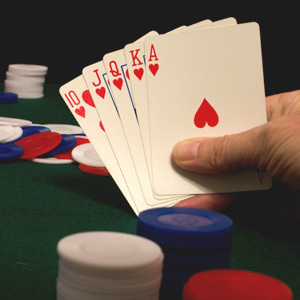
// Convert true color image to black & white using the Floyd-Steinberg algorithm
ImageEnView1.Proc.ConvertToBW_FloydSteinberg();
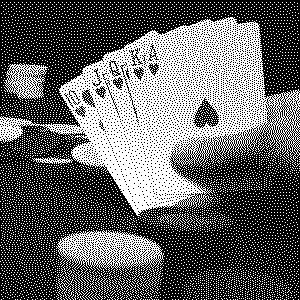
// Convert true color image to black & white using a local threshold algorithm (mean)
ImageEnView1.Proc.ConvertToBWLocalThreshold( 4, ietMean );
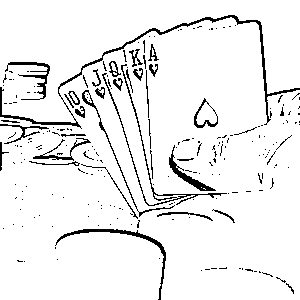
// Convert true color image to black & white using a local threshold algorithm (median)
ImageEnView1.Proc.ConvertToBWLocalThreshold( 4, ietMedian );
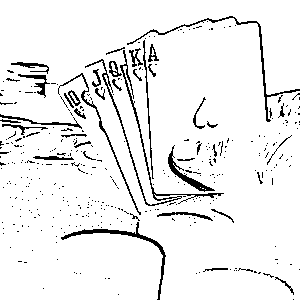
// Convert true color image to black & white using a local threshold algorithm (min/max mean)
ImageEnView1.Proc.ConvertToBWLocalThreshold( 4, ietMeanMinMax );
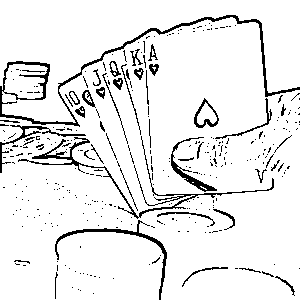
// Convert true color image to black & white with an ordered dithering method
ImageEnView1.Proc.ConvertToBWOrdered();
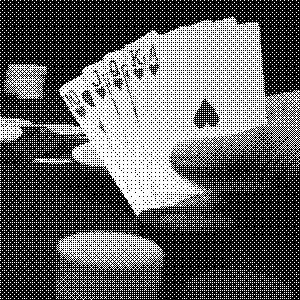
// Convert true color image to black & white using a thresholding algorithm
ImageEnView1.Proc.ConvertToBWThreshold( 128 );
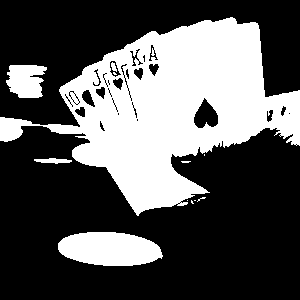
// Convert true color image to black & white using a thresholding algorithm (using the average level)
ImageEnView1.Proc.ConvertToBWThreshold( -1 );
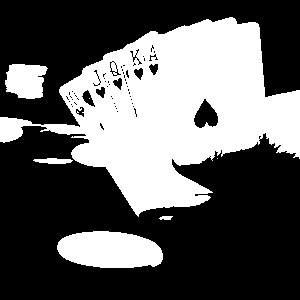
// Convert true color image to black & white using a thresholding algorithm (using maximum entropy)
ImageEnView1.Proc.ConvertToBWThreshold( -2 );
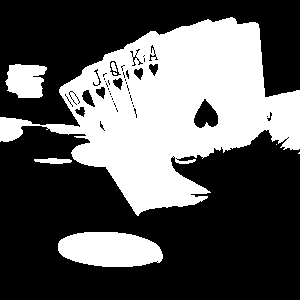
// All pixels < 128 wil be black >= 128 will be white
ImageEnView1.Proc.ConvertToBWThreshold(128);
// Auto calculate the threshold value as the average of all color levels
ImageEnView1.Proc.ConvertToBWThreshold(-1);
// Use maximum entropy algorithm
ImageEnView1.Proc.ConvertToBWThreshold(-2);
// Count blobs in an image
Test Image:
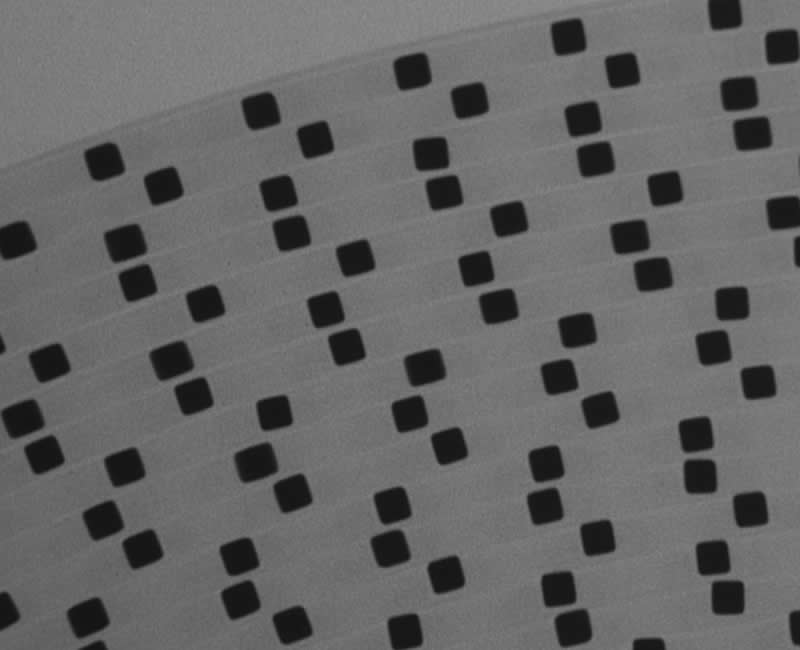
var
rects: TList;
i: integer;
r: TRect;
begin
ImageEnView1.Proc.ConvertToBWThreshold(80); // <= 80 = recommended parameter
rects := ImageEnView1.Proc.SeparateObjects(4, false);
// Needed to draw red rectangles around found objects!
ImageEnView1.Proc.ConvertTo24Bit();
for i := 0 to rects.Count-1 do
begin
r := PRect(rects[i])^;
// draw boxes
with ImageEnView1.IEBitmap.Canvas do
begin
Pen.Color := clRed;
Brush.Style := bsClear;
Rectangle( r.Left, r.Top, r.Right + 1, r.Bottom + 1 );
end;
dispose( PRect( rects[i] ));
end;
rects.Free();
ImageEnView1.Update();
ShowMessage('Found ' + IntToStr( rects.Count ) + ' objects');
End;
Result: 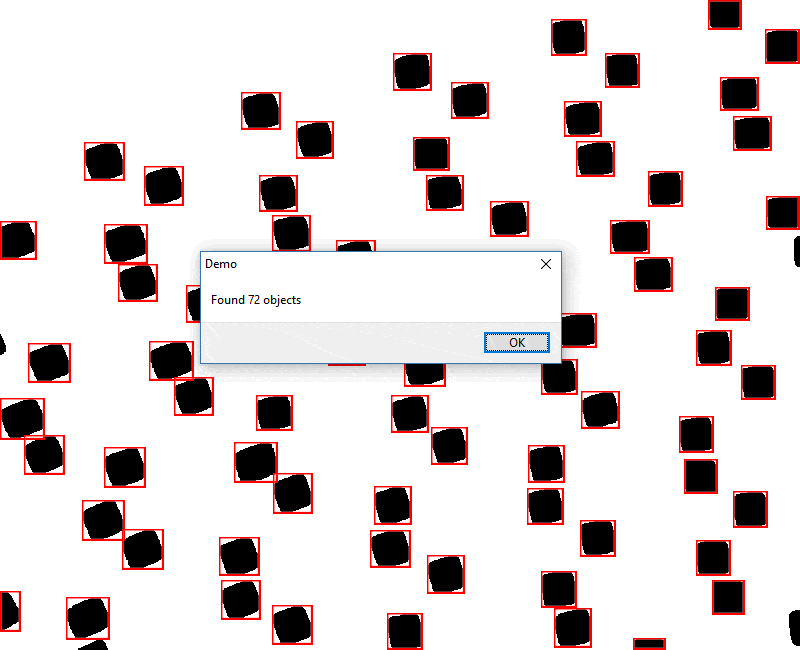
See Also
◼ConvertToBW_FloydSteinberg
◼ConvertToBWLocalThreshold
◼ConvertToBWOrdered
◼adaptiveThreshold