ImageEn, unit imageenview |
|
TImageEnView.PrepareTransition
Declaration
procedure PrepareTransition();
Description
PrepareTransition must be called prior to
running a transition effect.
It copies the currently displayed image to an internal buffer, which allows you to change current image (load, update, etc.) before starting the transition with
RunTransition.
| Demos\Display\Transitions\Transitions.dpr |
| Demos\Display\PanZoomEffects\PanZoomEffects.dpr |
Note:
◼An easier way to use display transition is just to set
TransitionEffect
◼PrepareTransition() is optional when showing a Pan-Zoom (iettPanZoom) transition
// Transition from Image1.jpg to Image2.jpg with "Random Points" transition
ImageEnView1.IO.LoadFromFile('D:\image1.jpg'); // Load initial image
ImageEnView1.PrepareTransition(); // Prepare the transition
ImageEnView1.IO.LoadFromFile('D:\image2.jpg'); // Load the next image (but does not display it)
ImageEnView1.RunTransition(iettRandompoints, 500); // Execute the transition
// Set properties for Word transition
ImageEnView1.TransitionParams.WordTransWord := 'BLAM';
ImageEnView1.TransitionParams.WordTransFontName := 'Arial';
ImageEnView1.TransitionParams.WordTransFontStyle := [fsBold];
// Prepare ImageEnView1 for a transition (e.g. so loading the image will not immediately update the display)
ImageEnView1.PrepareTransition();
// Load the next image
ImageEnView1.IO.LoadFromFile( GetNextImage() );
// Run the transition
ImageEnView1.RunTransition( iettRandomBoxesWithWord, 1000 );
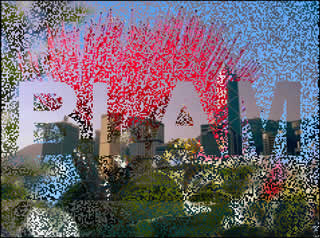
// Slide Transition from old.bmp to new.bmp ensuring that both are shown as the same height
procedure TForm1.btnCompareClick(Sender: TObject);
const
Display_Zoom = 100;
Center_Image = True;
var
bh: Integer;
iz: Double;
begin
// Get original image as transition start
ImageEnView1.IO.LoadFromFile( ExtractFilePath( Application.ExeName ) + 'old.bmp' );
ImageEnView1.Zoom := Display_Zoom;
if Center_Image then
ImageEnView1.CenterImage()
else
ImageEnView1.SetViewXY( 0, 0 );
bh := ImageEnView1.IEBitmap.Height;
iz := ImageEnView1.Zoom;
ImageEnView1.PrepareTransition();
// Load second image as transition result (Zoom to match display size of old image)
ImageEnView1.IO.LoadFromFile( ExtractFilePath( Application.ExeName ) + 'new.bmp' );
ImageEnView1.Zoom := iz / ( ImageEnView1.IEBitmap.Height / bh );
if Center_Image then
ImageEnView1.CenterImage()
else
ImageEnView1.SetViewXY( 0, 0 );
// Run the transition
ImageEnView1.TransitionParams.AlternativeStyle := True; // Include transition line
ImageEnView1.TransitionParams.WipeLineColor := clYellow;
ImageEnView1.RunTransition( iettRightLeft, 3000 ); // Wipe Right to Left
end;
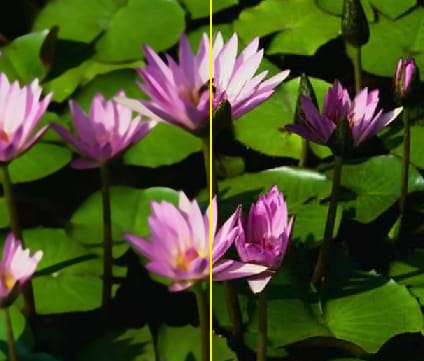
See Also
◼RunTransition
◼TransitionRunning
◼AbortTransition