Declaration
procedure smooth(dst: TIEVisionImage; smoothType: TIEVisionSmoothType = ievGAUSSIAN; param1: int32_t = 3; param2: int32_t = 0; param3: double = 0.0; param4: double = 0.0); overload; safecall;
procedure smooth(smoothType: TIEVisionSmoothType = ievGAUSSIAN; param1: int32_t = 3; param2: int32_t = 0; param3: double = 0.0; param4: double = 0.0); overload; safecall;
Description
Smooth the image.
First overload places the result into destination object.
Second overload updates the current image.
Also see:
Comparison of smoothing, blurring and noise reduction methods
Box Filter (ievBLUR_NO_SCALE/ievBLUR)
Performs a linear convolution filling the kernel with 1s. For ievBLUR the kernel is also normalized by its area, i.e. scaled by 1 / (param1 * param2).
To smooth different pixels with different-size box kernels, you can use the integral image that is computed using
integral.
Parameter | Description |
dst | Container for the destination image |
smoothType | Type of the smoothing |
param1 | Width of the blurring kernel box |
param2 | Height of the blurring kernel box (Specify 0 to use same value as param1) |
param3, param4 | Ignored |
Gaussian Blur (ievGAUSSIAN)
Convolves the image with a Gaussian kernel.
Parameter | Description |
dst | Container for the destination image |
smoothType | Type of the smoothing |
param1 | Width of the Gaussian kernel (Must be odd) |
param2 | Height of the Gaussian kernel (Must be odd, Specify 0 to use same value as param1) |
param3,param4 | Gaussian kernel standard deviation in X/Y direction (Specify param3/4 as 0 to compute from the kernel size) |
Median Blur (ievMEDIAN)
Blurs an image using a median filter.
Parameter | Description |
dst | Container for the destination image |
smoothType | Type of the smoothing |
param1 | Width and height of the aperture (Must be odd and greater than 1, e.g. 3, 5, 7...) |
param2,param3,param4 | Ignored |
Bilateral Filter (ievBILATERAL)
Applies a bilateral filter to an image. The Bilateral filter can reduce unwanted noise very well while keeping edges fairly sharp. However, it is very slow compared to most filters.
Parameter | Description |
dst | Container for the destination image |
smoothType | Type of the smoothing |
param1 | Diameter of each pixel neighborhood that is used during filtering. If specified as 0, it is computed from sigmaSpace (param4) |
param2 | Ignored |
param3 | Filter sigma in the color space. A larger value of the parameter means that farther colors within the pixel neighborhood will be mixed together, resulting in larger areas of semi-equal color |
param4 | Filter sigma in the coordinate space. A larger value of the parameter means that farther pixels will influence each other as long as their colors are close enough. When param1>0, it specifies the neighborhood size regardless of sigmaSpace. Otherwise, param1 is proportional to sigmaSpace |
Sigma values: For simplicity, you can set the 2 sigma values (param3/param4) to be the same. If they are small (e.g. < 10), the filter will not have much effect, whereas if they are large (> 150), they will have a very strong effect, making the image look "cartoonish".
Filter size: Large filters (param1 > 5) are very slow, so it is recommended to use param1=5 for real-time applications, and perhaps param1=9 for offline applications that need heavy noise filtering.
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
// Load test image
ImageEnView1.IO.LoadFromFile( 'D:\TestImage.jpg' );
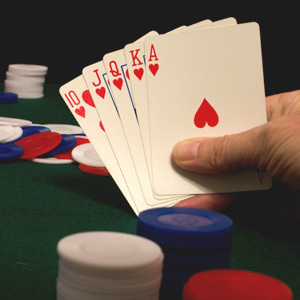
// Smooth image using Blur
ImageEnView1.IEBitmap.GetIEVisionImage().smooth( ievBLUR, 3, 3 );
ImageEnView1.Update();
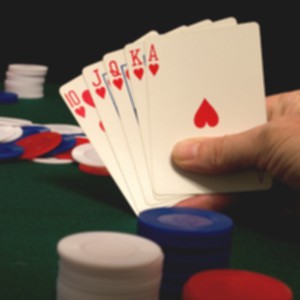
// Smooth image using Gaussian
// Parameter values specify the kernel size and must be odd
ImageEnView1.IEBitmap.GetIEVisionImage().smooth( ievGAUSSIAN, 3, 3 );
ImageEnView1.Update();
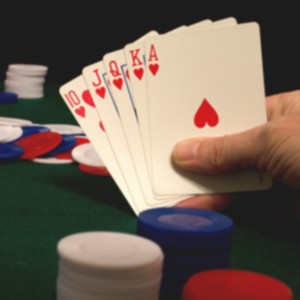
// Smooth image using Median
// Parameter values specify the aperture size and must be odd
ImageEnView1.IEBitmap.GetIEVisionImage().smooth( ievMEDIAN, 3 );
ImageEnView1.Update();
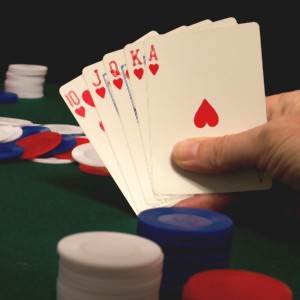
// Smooth image using Bilateral
// For Param3 and Param4, values in the range 30-100 are best
ImageEnView1.IEBitmap.GetIEVisionImage().smooth( ievBILATERAL, 5, 0, 30, 30 );
ImageEnView1.Update();
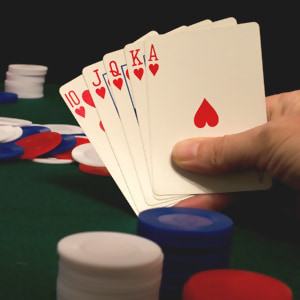