ImageEn, unit imageenproc |
|
TImageEnProc.AutoCrop
Declaration
function AutoCrop(Tolerance: Integer; Background: TRGB; DoCrop: Boolean = True; InnerCrop: Boolean = False; InnerCropThreshold: Double = 0.01): TRect;
function AutoCrop(Tolerance: Integer; Background: TColor; DoCrop: Boolean = True; InnerCrop: Boolean = False; InnerCropThreshold: Double = 0.01): TRect;
Description
Remove any bordering area of an image of a certain color.
if
InnerCrop = True, it will crop any border that contains
any of the specified color. This is a lossy process because valid area of the image will be lost.
Parameter | Description |
Tolerance | How closely we match the color to Background (0 to 255, where 0 matches only the specified color, and 255 would remove everything) |
Background | The border color to remove |
DoCrop | If False the image is not cropped, but the suggested area for cropping is returned as the result |
InnerCrop | If False, only rows/colums that contain all background are cropped. If true, rows/colums that contain any of the color will be cropped |
InnerCropThreshold | The minimum percentage of border color within a row/column to consider it border area, e.g. If 0.01, if more than 1% of pixels are background color then the row/column will be cropped. Lower values crop more aggressively (only use low values if you are sure the color does not exist in the image). Higher values crop less (and may leave background). Typical values are 0.001 - 0.02 |
Returns the area to crop or cropped.
If a border is not found the result will be a rect of (0, 0, w-1, h-1).
Note: AutoCrop() does
not consider the alpha channel
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
| Demos\IEVision\Stitcher\Stitcher.dpr |
Method Comparison
ImageEnView1.IO.LoadFromFile( 'D:\CropInput.png' );
// Image is 300x200 pixels
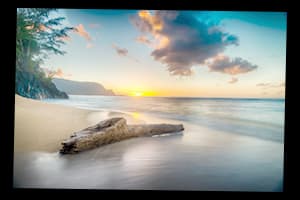
Outer Crop
ImageEnView1.Proc.AutoCrop( 0, clBlack, True );
// Image is now 274x184 pixels
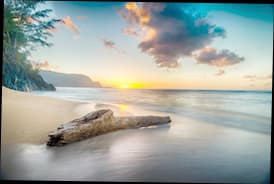
Inner Crop
ImageEnView1.Proc.AutoCrop( 0, clBlack, True, True, 0.01 );
// Image is now 262x176 pixels
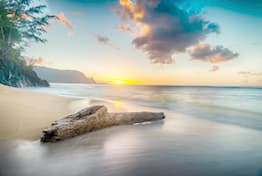
// Remove the black border from a scanned document
ImageEnView1.IO.Acquire();
ImageEnView1.Proc.AutoCrop(10, CreateRGB(0, 0, 0) );
// Crop an image, but not too tightly (leave a 10px margin)
rect := ImageEnView1.Proc.AutoCrop( 0, clWhite, False );
ImageEnView1.Proc.Crop( max( rect.Left - Crop_Margin, 0 ),
max( rect.Top - Crop_Margin, 0 ),
min( rect.Right + Crop_Margin, ImageEnView1.IEBitmap.Width ),
min( rect.Bottom + Crop_Margin, ImageEnView1.IEBitmap.Height ) );
// Load test image
ImageEnView1.IO.LoadFromFile( 'D:\TestImage.jpg' );
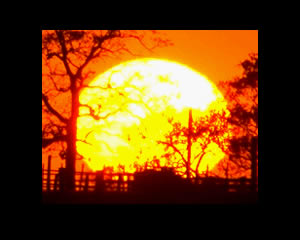
// Remove any black border from the image (with tolerance of 30)
ImageEnView1.Proc.AutoCrop( 30, clBlack );
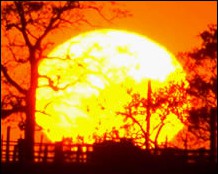
// Inner Cropping: Remove any black border even rows/columns that have non-black pixels
// Threshold is 0.01, so will crop any rows/columns that have more than 0.1% black pixels
ImageEnView1.Proc.AutoCrop( 0, clBlack, True, True, 0.001 );
See Also
◼AutoCrop2◼CropTransparentBorder◼Crop