ImageEn, unit imageenproc |
|
TImageEnProc.CastColorRange
Declaration
procedure CastColorRange(BeginColor, EndColor, CastColor: TRGB); overload;
procedure CastColorRange(BeginColor, EndColor, CastColor: TColor); overload;
procedure CastColorRange(OldColor, NewColor: TRGB; Tolerance: Integer); overload;
Description
Set all colors in the range BeginColor to EndColor to CastColor.
Note:
◼Ensure
BeginColor <= EndColor, i.e.
BeginColor is a darker color (Black is 0,0,0) and
EndColor a lighter color (white is 255, 255, 255).
◼To cast in a flood fill manner, use
CastColor
◼If the image
PixelFormat is not ie24RGB, it will be converted
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
// Change all gray levels from 50 to 100 to black
var
BeginColor, EndColor, CastColor: TRGB;
begin
BeginColor := CreateRGB(50, 50, 50);
EndColor := CreateRGB(100, 100, 100);
CastColor := CreateRGB(0, 0, 0);
ImageEnView1.Proc.CastColorRange(BeginColor, EndColor, CastColor);
end;
// When the user clicks the image turn all image pixels of a similar color to white
procedure TForm1.ImageEnView1MouseDown(Sender: TObject; Button: TMouseButton;
Shift: TShiftState; X, Y: Integer);
var
clickColor: TRGB;
begin
if fColorCasting then
begin
clickColor := ImageEnView1.IEBitmap.Pixels[ ImageEnView1.XScr2Bmp(x), ImageEnView1.YScr2Bmp(y) ];
ImageEnView1.Proc.CastColorRange( clickColor, CreateRGB(255, 255, 255), 10);
end;
end;
// Convert a 32bit PNG with 8bit alpha to a BMP with fuschsia background (e.g. for Delphi bitmaps glyphs)
const
Anti_Aliasing_Color = $00C9C9C9; // Light gray
begin
ImageEnView1.IO.LoadFromFile( 'D:\CameraIcon.png' );
ImageEnView1.IEBitmap.RemoveAlphaChannel( True, Anti_Aliasing_Color ); // Merge alpha with anti-aliasing color
ImageEnView1.Proc.CastColorRange( Anti_Aliasing_Color, Anti_Aliasing_Color, clFuchsia ); // Convert background to fuchsia
ImageEnView1.IO.SaveToFile( 'D:\CameraGlyph.bmp' );
end;
// Load test image
ImageEnView1.IO.LoadFromFile( 'D:\TestImage.jpg' );
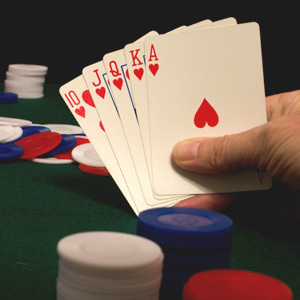
// Change all dark values (from dark gray to black) to black
ImageEnView1.Proc.CastColorRange( CreateRGB(0, 0, 0),
CreateRGB(100, 100, 100),
CreateRGB(0, 0, 0));
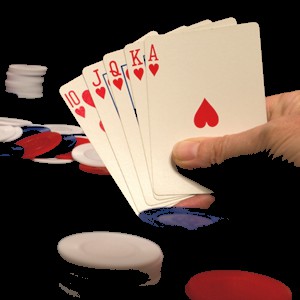
See Also
◼CreateRGB
◼TRGB2TColor
◼TColor2TRGB