ImageEn, unit iexHelperFunctions |
|
TIEBitmapHelper.FindObjects
Declaration
function FindObjects(const Classifier: string; MergeOverlapping: Boolean = True; SpeedDivisor: Integer = 3): TIERectArray; overload;
function FindObjects(const Classifiers: Array of String; MergeOverlapping: Boolean = True; SpeedDivisor: Integer = 3): TIERectArray; overload;
Description
A shortcut method that creates a
TIEVisionObjectsFinder object, adds the specified
classifier(s) and calls
findIn.
Parameter | Description |
Classifiers | The classifiers to create and add. You can specify in-built classifiers or classifier filename(s) |
MergeOverlapping | If you specify multiple classifiers, they may return the same rects, so it is best to merge any overlapping ones |
SpeedDivisor | Speeds up processing (at the cost of accuracy) by reducing the size of the analyzed image, specify 1 to analyze the image at current size |
Note:
◼You must add the iexHelperFunctions unit to your uses clause
◼Delphi/C++ 2005 or newer is required to use helper classes
◼You can draw the rects to a canvas using
DrawRects
◼You can create your own
classifiers using
TIEVisionCascadeClassifierTrainer
◼Object finding requires
IEVision. You will need to
register it before calling the method
Method Behaviour
The following call:
rects := ImageEnView1.IEBitmap.FindObjects([ IEVC_FRONTAL_FACE_DEFAULT, IEVC_FRONTAL_FACE_ALT, IEVC_FRONTAL_FACE_ALT_2, IEVC_FRONTAL_FACE_ALT_TREE ], True, 3 );
Is the same as calling:
objectsFinder := IEVisionLib.createObjectsFinder();
objectsFinder.addClassifier('face detector 1', IEVisionLib.createCascadeClassifier(IEVC_FRONTAL_FACE_DEFAULT));
objectsFinder.addClassifier('face detector 2', IEVisionLib.createCascadeClassifier(IEVC_FRONTAL_FACE_ALT_TREE));
objectsFinder.addClassifier('face detector 3', IEVisionLib.createCascadeClassifier(IEVC_FRONTAL_FACE_ALT));
objectsFinder.addClassifier('face detector 4', IEVisionLib.createCascadeClassifier(IEVC_FRONTAL_FACE_ALT_2));
objectsFinder.setDivisor( 3 );
objectsFinder.findIn(ImageEnView1.IEBitmap.GetIEVisionImage());
rects := objectsFinder.mergeAllRects(); // merge intersecting rectangles
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
// Find Faces in image
rects := ImageEnView1.IEBitmap.FindObjects([ IEVC_FRONTAL_FACE_DEFAULT, IEVC_FRONTAL_FACE_ALT, IEVC_FRONTAL_FACE_ALT_2, IEVC_FRONTAL_FACE_ALT_TREE, IEVC_PROFILE_FACE ], True, 1 );
// Draw rects to image
for i := 0 to Length(rects) - 1 do
begin
r := rects[i];
with ImageEnView1.IEBitmap.Canvas do
begin
Pen.Width := 2;
Pen.Color := clRed;
Brush.Style := bsClear;
Rectangle( r.x, r.y, r.x + r.width, r.y + r.width );
end;
end;
ImageEnView1.Proc.TextOut( Align_Text_Near_Left, Align_Text_Near_Top, Format( 'Found: %d', [ Length(rects) ]), 'Arial', 12, Text_Color, [fsBold] );
ImageEnView1.Update();
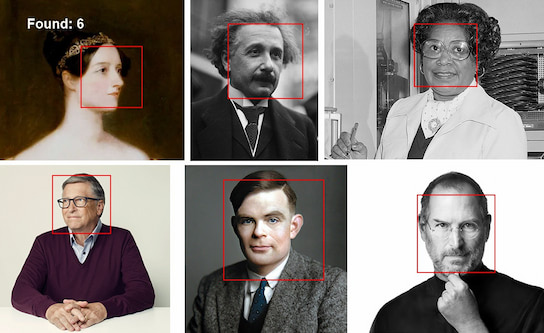
// Load classifiers from file
rects := ImageEnView1.IEBitmap.FindObjects([ 'D:\Classifiers\haarcascade_eyepair.xml',
'D:\Classifiers\haarcascade_mouth.xml',
'D:\Classifiers\haarcascade_nose.xml' ], False, 1 );
// loop among rectangles
for i := 0 to Length( rects ) - 1 do
begin
// Add rectangles around objects
ImageEnView1.LayersAdd( iesRectangle, rects[i], clRed, 2 );
// Copy object image to a TImageEnMView
ImageEnMView1.SetImageRect( ImageEnMView1.AppendImage(), ImageEnView1.IEBitmap, rects[i].Left, rects[i].Top, rects[i].Right, rects[i].Bottom );
end;