Declaration
function StitchImages(Dest: TIEBitmap; StitchMode: TIEVisionStitcherMode = ievSTITCH_PANORAMA; ConfidenceTreshold: Double = 1.0; TryUseGPU: Boolean = False; CropImage: Boolean = False; CropThreshold: Double = 0.01): TIEVisionStitchingStatus;
Description
A shortcut method that creates a
TIEVisionStitcher object and calls
stitch to join multiple images into a single panoramic image.
Parameter | Description |
Dest | The bitmap that will be assigned the resulting image (must be created before calling) |
StitchMode | The stitching mode to use (ievSTITCH_PANORAMA for photos or ievSTITCH_SCAN for scanned images) |
ConfidenceTreshold | The minimum level of confidence in the stitched result that is accepted |
TryUseGPU | If supported, the GPU will be used to improve performance |
CropImage | If enabled, the resulting image is cropped to remove any black null area. Note: This is a lossy process because valid area of the image will be lost |
CropThreshold | The minimum percentage of black within a row/column to consider it border area, e.g. If 0.01, if more than 1% of pixels are black then the row/column will be cropped. Lower values crop more aggressively (only use low values if you are sure the color does not exist in the image). Higher values crop less (and may leave background). Typical values are 0.001 - 0.02 |
Result is ievSSOK if the stitching is successful, otherwise it will be an error type: ievSSERR_NEED_MORE_IMGS, ievSSERR_HOMOGRAPHY_EST_FAIL, ievSSERR_CAMERA_PARAMS_ADJUST_FAIL
When the
TIEMultiBitmap is attached to a
TImageEnMView:
◼If
Checkboxes are enabled, only checked images will be included. An exception is raised if there are no checked items
◼If
StoreType is not ietNormal, an attempt is made to load full quality images from file. An exception is raised if the files cannot be accessed
Note:
◼You must add the iexHelperFunctions unit to your uses clause
◼Delphi/C++ 2005 or newer is required to use helper classes
◼Stitching requires
IEVision. You will need to
register it before calling the method
◼If Dest is attached to a
TImageEnView, it will automatically call
Update
◼Stitching is randomized each time StitchImages is called, so you may need to call it multiple times to get a desirable result
◼If you encountering failures (particularly
ievSSERR_NEED_MORE_IMGS), ensure that your source images contain enough overlapping content. Try
lowering the threshold to be more accepting of results
◼ievSSERR_CAMERA_PARAMS_ADJUST_FAIL typically occurs when trying to stitch scanned images in panoramic mode, so ensure you set ievSTITCH_SCAN mode when
creating the stitcher. Also try increasing the
registration resolution
◼Also see
JoinBitmaps which allows joining of two images with overlap support
Method Behaviour
The following call:
ImageEnMView1.IEMBitmap.StitchImages( ImageEnView1.IEBitmap, ievSTITCH_PANORAMA, 1.0, True, True, 0.01 );
Is the same as calling:
images := IEVisionLib.createVectorImageRef();
for i := 0 to ImageEnMView1.ImageCount - 1 do
begin
bmp := ImageEnMView1.GetTIEBitmap( i, True );
images.Push_Back( IEVisionLib.createImage( bmp.GetIEVisionImage() ));
ImageEnMView1.ReleaseBitmap( i, False );
end;
// Stitch images
stitcher := IEVisionLib.createStitcher( True, ievSTITCH_PANORAMA );
stitcher.setPanoConfidenceThresh( 1.0 );
pano := sticher.stitch( images, status, True );
if status = ievSSOK then
begin
ImageEnView1.IEBitmap.AssignIEVisionImage( pano );
ImageEnView1.Proc.AutoCrop( 0, clBlack, True, True, 0.0001 ); // Crop any rows/columns that have more than 0.1% black pixels
end;
// Stitch all the images in ImageEnMView1 and display the result in ImageEnView1
ShowTempHourglass();
if ImageEnMView1.IEMBitmap.StitchImages( ImageEnView1.IEBitmap, ievSTITCH_PANORAMA ) = ievSSOK then
ImageEnView1.Fit( False );
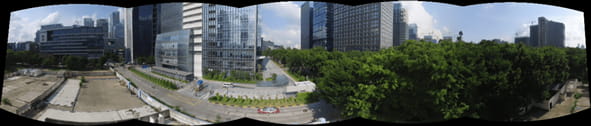
// Same as above but with cropping enabled
ShowTempHourglass();
if ImageEnMView1.IEMBitmap.StitchImages( ImageEnView1.IEBitmap, ievSTITCH_PANORAMA, 1.0, True, True, 0.01 ) = ievSSOK then
ImageEnView1.Fit( False );

// Stitch 3 scanned image files
mbmp := TIEMultiBitmap.Create();
mbmp.FillFromList( ['D:\image1.png', 'D:\image2.png', 'D:\image3.png'] );
mbmp.StitchImages( ImageEnView1.IEBitmap, ievSTITCH_SCAN, 0.7 );
mbmp.Free;