ImageEn, unit imageenproc |
|
TImageEnProc.RotateAndCrop
Declaration
procedure RotateAndCrop(Angle: Double; AntiAliasMode: TIEAntialiasMode = ierFast; Rate: Double = 1.3; CropAlgorithm: TIECropAlgorithm = iecaSkewedDocument);
Description
Rotates the image by the specified angle and crops the borders of the original bitmap. It is useful to deskew an image if you know the rotation angle.
Parameter | Description |
Angle | Rotation angle. Must be in the range -180 and +180 degrees (specified as counter-clockwise, i.e. RotateAndCrop(45) means rotated 45° left) |
AntiAliasMode | Specifies the anti-aliasing algorithm that is used to improve rotation quality |
Rate | Specifies the approximate quotient of resulting image width and height. For example a landscape image would typically be 1.3, whereas a portrait image would be 0.75. This value is only needed when Angle is +/-45 or +/-135 and CropAlgorithm is iecaSkewedDocument |
CropAlgorithm | Generally both cropping algorithms will give similar results, but specifying an appropriate algorithm can improve results at higher rotation levels |
Crop Algorithm
Value | Description |
iecaSkewedDocument | Works best with documents requiring rotation due to scanning skew (not lined up correctly when scanning) or a previous rotation. In this case the image will generally already have unwanted null/white area which should be be trimmed |
iecaAngledPhoto | Works best with photos requiring rotation due camera skew (the camera is not quite horizontal). In this case the image looks valid, but the horizon is not horizontal. After rotation the image will have new null/background area, which we want to trim |
Skewed Document Example
This is an image that has been incorrectly rotated and so is skewed and has unwanted white space (which is the same as a document that was not lined up when scanning):
You can determine the required rotation by calling:
r := ImageEnView1.Proc.SkewDetection();
Then you can rotate the image using
ImageEnView1.Proc.Rotate(r), but you get more white space:
The gray border (which is part of the original bitmap) is rotated with the image. To remove that border you must instead use
ImageEnView1.Proc.RotateAndCrop(r), which generates:
Skewed Photo Example
This is a common image from a digital camera that was not held correctly. All the image is valid but it is skewed and needs to be lined up (e.g. using the
RotateTool):
If we were to rotate to level out the horizon, we would call
ImageEnView1.Proc.Rotate( 5 ), but this will generate white space in the null areas:
To rotate without leaving any border, we must call
ImageEnView1.Proc.RotateAndCrop( 5 ), which generates:
Note:
◼A UI for this is available to your users in the
Image Processing dialog
◼To crop and rotate an ROI, use
Crop
| Demos\ImageEditing\RotateAndCrop\RotateAndCrop.dpr |
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
// Detect the skew in an image and automatically rotate and crop it
angle := ImageEnView1.Proc.SkewDetection( ImageEnView1.IEBitmap.Width div 4, 30, 0.1, false );
ImageEnView1.Proc.RotateAndCrop( angle );
// Rotate and crop a landscape document by 45 degrees
ImageEnView1.Proc.RotateAndCrop( 45, ierBicubic, 1.3 );
// Rotate and crop a portrait document by 45 degrees
ImageEnView1.Proc.RotateAndCrop( 45, ierBicubic, 0.75 );
// Rotate and crop a digital photo by 5 degrees
ImageEnView1.Proc.RotateAndCrop( 5, ierBicubic, 0, iecaAngledPhoto );
// Image tests
ImageEnView1.IO.LoadFromFile( 'D:\TestImage.jpg' );
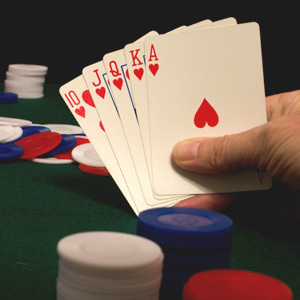
// Rotate the image 15° counter-clockwise and crop to maintain only image area
ImageEnView1.Proc.RotateAndCrop( 15, ierBicubic, 0, iecaAngledPhoto );
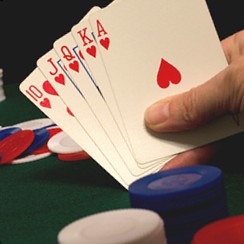