Declaration
TIEVisionImage = interface(TIEVisionBase)
Description
An interface to handle raster images (or
matrices) of the type required by IEVision.
It is possible to perform basic input/output operations and several image processing tasks. You can also connect a TIEVisionImage to a
TIEBitmap, sharing the same content.
To convert a TIEBitmap to a TIEVisionImage use
GetIEVisionImage:
vizImage := ImageEnView1.IEBitmap.GetIEVisionImage();
To convert a TIEVisionImage to a TIEBitmap use
AssignIEVisionImage:
ImageEnView1.IEBitmap.AssignIEVisionImage( vizImage );
For TBitmap you can
assign it first to a
TIEBitmap.
// Create empty image
image := IEVisionLib.createImage();
// Create an image from "input.jpg", resize to 100x100 and save as "output.jpg"
image := IEVisionLib.createImage('input.jpg');
image.resize(100, 100); // default interpolation = linear
image.save('output.jpg');
// Create an image of 1000x1000x24 bit
image := IEVisionLib.createImage(1000, 1000, ievUINT8, 3);
// Create an image from the TIEBitmap of a TImageEnView (sharing the content)
ImageEnView1.IEBitmap.Origin := ieboTOPLEFT;
image := IEVisionLib.createImage(ImageEnView1.IEBitmap.Width, ImageEnView1.IEBitmap.Height,
ievUINT8, 3, ImageEnView1.IEBitmap.Rowlen,
ImageEnView1.IEBitmap.ScanLine[0]);
// Same as previous code
image := ImageEnView1.IEBitmap.GetIEVisionImage();
var
images: TIEVisionVectorImageRef;
i: Integer;
pano: TIEVisionImage;
status: TIEVisionStitchingStatus;
begin
images := IEVisionLib.createVectorImageRef();
for i := 1 to 6 do
images.push_back(IEVisionLib.createImage('image' + IntToStr( i ) + '.jpg'));
pano := IEVisionLib.createStitcher().stitch(images, status);
if status = ievSSOK then
ImageEnView1.IEBitmap.AssignIEVisionImage(pano);
end;
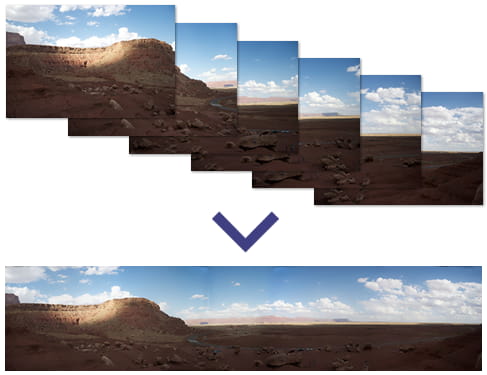
General
Image Analysis
Image Processing
Matrix Methods
Pixel Access
See Also
◼createImage