TIEPolylineLayer.AddCurvePoints
Declaration
procedure AddCurvePoints(StartAngle, SweepAngle: Double; EllipseWidth, EllipseHeight: Double; PtCount: Integer = 0); overload;
procedure AddCurvePoints(RadiusMult: Double; EndPoint: TPoint; PtCount: Integer = 0); overload;
Description
Add points to the end of a polyline in a curve.
Overload 1:
Parameter | Description |
StartAngle | The point on the imaginary circle where we start (e.g. 0 starts at 3 on a clock face, -90 starts at 6 o'clock) |
SweepAngle | The number of degrees to sweep. Negative values sweep clockwise (e.g. 360 would create a full circle, whereas is -180 would create a clockwise half circle) |
EllipseWidth | The width of the imaginary circle in the range 0 - 1000 (e.g. 500 would make a circle half the width of the layer) |
EllipseHeight | The width of the imaginary circle in the range 0 - 1000 (e.g. 500 would make a circle half the width of the layer) |
PtCount | The number of points to add (if zero, it is calculated based on LayerCurveQuality) |
Overload 2:
Parameter | Description |
RadiusMult | Specify a multiplier to increase or decrease the radius of the curve. 1: Creates a curve of an exact half circle. >1 creates a shallower curve. <1 creates a bulging curve. Pass as negative to sweep clockwise |
EndPoint | The location of the final point in the range 0 - 1000 (e.g. 500, 500 would put the final point in the center of the layer) |
PtCount | The number of points to add (if zero, it is calculated based on LayerCurveQuality) |
Note:
◼The curve will start at the previous point, so will fail if the polyline layer does not contain points
◼You can also convert a line to a curve by holding down the alt key when editing a polyline (i.e. when mlEditLayerPoints is set for
MouseInteractLayers)
◼For best quality, PtCount should be less than SweepAngle. Around SweepAngle/4 is typical.
// Add a 90 deg. curve from 6 o'clock to 3 o'clock (using overload 1)
polyLayer := TIEPolylineLayer( ImageEnView1.CurrentLayer );
polyLayer.AddPoint( 500, 500 );
polyLayer.AddCurvePoints( 270, 90, 500, 500, 20 );
ImageEnView1.Update();
// Add a 270 deg. curve from 6 o'clock to 3 o'clock (using overload 1)
polyLayer := TIEPolylineLayer( ImageEnView1.CurrentLayer );
polyLayer.AddPoint( 500, 500 );
polyLayer.AddCurvePoints( 270, -90, 500, 500, 20 );
ImageEnView1.Update();
// Draw a red heart
ImageEnView1.LayersAdd( ielkPolyline, 300, 300, 400, 400 );
With TIEPolylineLayer( ImageEnView1.CurrentLayer ) do
begin
AddPoint( 100, 500 );
AddCurvePoints( -1, Point( 500, 200 ), 20 );
AddCurvePoints( -1, Point( 900, 500 ), 20 );
AddPoint( 500, 1000 );
LineWidth := 0;
FillColor := clRed;
PolylineClosed := True;
end;
ImageEnView1.Update();
// Draw a half circle curving downward (using overload 2)
TIEPolylineLayer( ImageEnView1.CurrentLayer ).AddPoint( 0, 500 );
TIEPolylineLayer( ImageEnView1.CurrentLayer ).AddCurvePoints( 1, Point( 1000, 500 ));
// Draw a half circle curving upward (using overload 2)
TIEPolylineLayer( ImageEnView1.CurrentLayer ).AddPoint( 0, 500 );
TIEPolylineLayer( ImageEnView1.CurrentLayer ).AddCurvePoints( -1, Point( 1000, 500 ));
// Draw a shallow smiley face (using overload 2)
TIEPolylineLayer( ImageEnView1.CurrentLayer ).AddPoint( 0, 500 );
TIEPolylineLayer( ImageEnView1.CurrentLayer ).AddCurvePoints( 3, Point( 1000, 500 ));
// Draw a shallow sad face (using overload 2)
TIEPolylineLayer( ImageEnView1.CurrentLayer ).AddPoint( 0, 500 );
TIEPolylineLayer( ImageEnView1.CurrentLayer ).AddCurvePoints( -3, Point( 1000, 500 ));
// Draw a bulging curve downward (using overload 2)
TIEPolylineLayer( ImageEnView1.CurrentLayer ).AddPoint( 0, 500 );
TIEPolylineLayer( ImageEnView1.CurrentLayer ).AddCurvePoints( 0.5, Point( 1000, 500 ));
// Draw a bulging curve upward (using overload 2)
TIEPolylineLayer( ImageEnView1.CurrentLayer ).AddPoint( 0, 500 );
TIEPolylineLayer( ImageEnView1.CurrentLayer ).AddCurvePoints( 0.5, Point( 1000, 500 ));
// Draw a blue raindrop (using overload 2)
ImageEnView1.LayersAdd( ielkPolyline, 600, 300, 400, 400 );
With TIEPolylineLayer( ImageEnView1.CurrentLayer ) do
begin
AddPoint( 100, 500 );
AddCurvePoints( 0.1, Point( 800, 500 ), 30 );
AddPoint( 500, 0 );
LineWidth := 0;
FillColor := clSkyBlue;
PolylineClosed := True;
end;
ImageEnView1.Update();
// Curve from 270 (6 o'clock) anti-clockwise to 180 (9 o'clock)
StartAngle := 270;
EndAngle := 90;
SweepAngle := EndAngle - StartAngle + 360;
if SweepAngle >= 360 then
SweepAngle := SweepAngle - 360;
TIEPolylineLayer( ImageEnView1.CurrentLayer).AddCurvePoints( StartAngle, SweepAngle, 1000, 1000, 30 );
// Draw a pink balloon
ImageEnView1.LayersAdd( ielkPolyline, 100, 100, 600, 800 );
With TIEPolylineLayer( ImageEnView1.CurrentLayer ) do
begin
AddPoint( 500, 500 );
AddCurvePoints( 0.95, Point( 500, 0 ));
AddCurvePoints( 0.95, Point( 500, 500 ));
AddPoint($FFFEE, $FFFEE); // End shape and close polyline
AddPoint( 500, 500 );
AddPoint( 520, 517 );
AddPoint( 480, 517 );
AddPoint($FFFEE, $FFFEE); // End shape and close polyline
AddPoint( 500, 517 );
AddCurvePoints( 3, Point( 500, 690 ));
AddCurvePoints( -3, Point( 510, 850 ));
AddCurvePoints( 3, Point( 500, 1000 ));
AddPoint($FFFFF, $FFFFF); // End polyline (optional at end)
LineWidth := 2;
FillColor := $00921CF0;
PolylineClosed := False;
end;
ImageEnView1.Update();
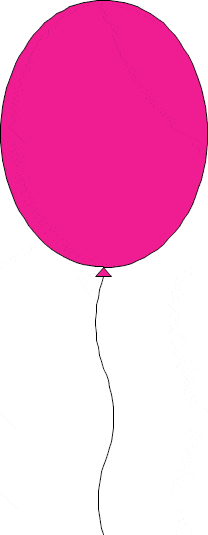
See Also
◼InsertCurvePoints
◼AddPoint
◼Points