Declaration
property MouseInteractLayers: TIEMouseInteractLayers;
Description
An extension to
MouseInteractGeneral for mouse activities related to layer editing and creation.
Value | Description |
mlClickCreateAngleLayers | Click three points to create a TIEAngleLayer (Notes 1,2). Click Esc to cancel insertion |
mlClickCreateLineLayers | Click start and end points to create a TIELineLayer (Notes 1,2). Click Esc to cancel insertion |
mlClickCreatePolylineLayers | Click multiple points to create a TIEPolylineLayer (Notes 1,2). Click Enter, Double-click, or click near the start point to complete the polyline. Use LayersAutoClosePolylines to determine whether the polyline is closed (i.e. becomes a polygon). Click Esc to cancel insertion, or DEL to delete the last added point |
mlDrawCreatePolylineLayers | Click and drag to freehand draw a TIEPolylineLayer (Notes 1,2) |
mlCreateImageLayers | Click and drag the mouse to create a TIEImageLayer (Notes 1,3) |
mlCreateShapeLayers | Click and drag the mouse to create a TIEShapeLayer (Notes 1,3) |
mlCreateLineLayers | Click and drag the mouse to create a TIELineLayer (Notes 1,3) |
mlCreateAngleLayers | Click and drag the mouse to create a TIEAngleLayer (Notes 1,3) |
mlCreatePolylineLayers | Click and drag the mouse to create a TIEPolylineLayer (Notes 1,3). This creates area of the layer, you will need to add points |
mlCreateTextLayers | Click and drag the mouse to create a TIETextLayer (Notes 1,3) |
mlEditLayerPoints | Click and drag points of a TIELineLayer or TIEPolylineLayer to move them. Click on the polyline to add a point, Alt key to convert a point to a curve, Ctrl+click or delete key to remove a point. Hold Alt key, then click on a line layer and drag to convert to a curve (Note 2,4) |
mlMoveLayers | User can move layers |
mlResizeLayers | User can resize layers using grips. Also see: LayersFixSizes |
mlRotateLayers | User can rotate layers by dragging the rotation grip (if loShowRotationGrip is enabled), otherwise clicking and dragging anywhere outside the layer (Notes 2,5). Also see: LayersFixRotations |
Note:
1. If you are unable to create layers on the background, ensure that ImageEnView1.Layers[0].
Selectable = False
2. Hold Shift key to lock line angles to
LayersRotateStep
3. If
loStampMode is specified for
LayerOptions, a single click will add the layer
4. Hold Alt key to convert point to a curve. Alt+Shift will give an exact half circle. Use
LayersCurvePoints to specify how many points are used for a curve
5. Use
mlRotateLayers in layer applications, and
miRotateTool in other (single layer) applications
Other Notes:
◼Mouse interaction options are split into two properties:
MouseInteractLayers and
MouseInteractGeneral. Items of
MouseInteractLayers relate only to layer editing and creation, whereas
MouseInteractGeneral are all remaining options (selection, view, measurement and image editing functions)
◼Multiple interactions can be specified, but activities that are not mutually compatible will be excluded
◼Setting
MouseInteractLayers, will also remove incompatible items from
MouseInteractGeneral
◼Use
LayersResizeAspectRatio to control aspect ratio locking behavior
◼Layer interactions cannot be set when the
PdfViewer is enabled unless
AllowLayers is set
Default: []
Usage Notes
You can only select one of the following:
mlCreateImageLayers, mlCreateShapeLayers, mlCreateLineLayers, mlCreatePolylineLayers, mlCreateAngleLayers, mlCreateTextLayers,
mlClickCreateLineLayers, mlClickCreatePolylineLayers, mlDrawCreatePolylineLayers, mlClickCreateAngleLayers
The following are often used in combinations with others:
miZoom, miSmoothZoom, miDblClickZoom, miMovingScroll, mlEditLayerPoints,
mlMoveLayers, mlResizeLayers, mlRotateLayers (can be used with any of the
miSelect* methods, except miSelectZoom). When miSelect and mlMoveLayer are specified together, then movement is only possible by clicking and dragging the edge of the current layer,
To use mlRotateLayers in combination with layer creation interactions (mlCreate*Layers),
loShowRotationGrip must be included for
LayerOptions
if mlEditLayerPoints and mlResizeLayers are used in combination, point layers will only be resizable by dragging their points
| Demos\Other\MouseInteract\MouseInteract.dpr |
| Demos\LayerEditing\RotateLayers\RotateLayers.dpr |
| Demos\LayerEditing\Layers_AllTypes\Layers.dpr |
| Demos\LayerEditing\Layers_Lines\Layers.dpr |
// Allow users to create image layers. Prompt for an image file after selection
ImageEnView1.LayerOptions := ImageEnView1.LayerOptions + [ loAutoPromptForImage ];
ImageEnView1.MouseInteractLayers := [ mlCreateImageLayers ];
// Allow user to move and resize layers (allow multiple layer selection and ensure masks are moved with layers)
ImageEnView1.LayerOptions := ImageEnView1.LayerOptions + [ loAllowMultiSelect, loAutoSelectMask ];
ImageEnView1.MouseInteractLayers := [ mlMoveLayers, mlResizeLayers ];
A selected text layer with resize grips:
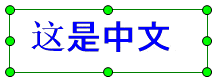
Four selected layers with resize grips:
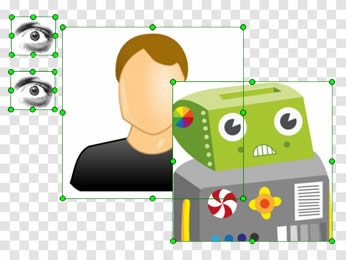
// Allow user to rotate layers (allow multiple layer selection and ensure masks are moved with layers)
ImageEnView1.LayerOptions := ImageEnView1.LayerOptions + [ loAllowMultiSelect, loAutoSelectMask ];
ImageEnView1.MouseInteractLayers := [ mlRotateLayers ];
// Allow the user to create, size and rotate red arrows
ImageEnView1.LayerOptions := ImageEnView1.LayerOptions + [ loShowRotationGrip ];
ImageEnView1.MouseInteractLayers := [ mlCreateLineLayers, mlMoveLayers, mlResizeLayers, mlRotateLayers ];
ImageEnView1.LayerDefaults.Clear();
ImageEnView1.LayerDefaults.Values[ IELP_LineColor ] := 'clRed';
ImageEnView1.LayerDefaults.Values[ IELP_LineWidth ] := '6';
ImageEnView1.LayerDefaults.Values[ IELP_LineShapeSize ] := '20';
ImageEnView1.LayerDefaults.Values[ IELP_LineStartShape ] := '1';
ImageEnView1.LayerDefaults.Values[ IELP_Rotate ] := '235';
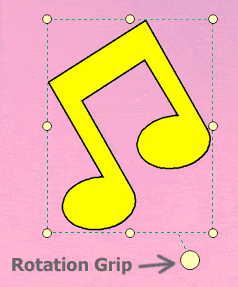
// Allow user to create star shapes at preferred aspect ratio
ImageEnView1.LayersResizeAspectRatio := iearAlways;
IEGlobalSettings().DefaultLayerShape := iesStar5;
ImageEnView1.MouseInteractLayers := [ mlCreateShapeLayers ];
// Allow user to draw a polygon
ImageEnView1.LayersAutoClosePolylines := iecmAlways;
ImageEnView1.MouseInteractLayers := [ mlClickCreatePolylineLayers ];
// Allow users to create and edit a polyline
ImageEnView1.LayersAutoClosePolylines := iecmManual;
ImageEnView1.MouseInteractLayers := [ mlClickCreatePolylineLayers, mlEditLayerPoints ];
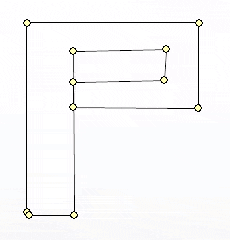
// Allow users to edit a polyline. Hold Alt key to turn line into a curve
ImageEnView1.MouseInteractLayers := [ mlEditLayerPoints ];
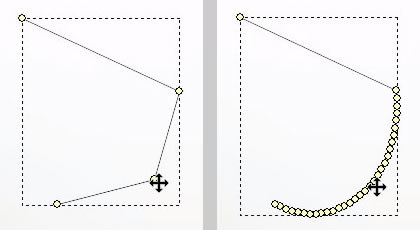
// Allow users to draw rulers (lines with measurement values)
With ImageEnView1.LayerDefaults do
begin
Clear();
Values[ IELP_RulerMode ] := IntToStr( ord( iermLabel ));
Values[ IELP_RulerUnits ] := IntToStr( ord( ieuCentimeters ));
Values[ IELP_LabelPosition ] := IntToStr( ord( ielpAutoAbove ));
Values[ IELP_LineStartShape ] := IntToStr( ord( ieesBar ));
Values[ IELP_LineEndShape ] := IntToStr( ord( ieesBar ));
Values[ IELP_LineWidth ] := '2';
end;
IEGlobalSettings().MeasureDecimalPlaces := 1;
ImageEnView1.MouseInteractLayers := [ mlClickCreateLineLayers, mlEditLayerPoints ];
// Allow users to create text layers. Start in text editing mode (user can immediately begin typing text)
ImageEnView1.LayerOptions := ImageEnView1.LayerOptions + [ loAutoTextEditing ];
ImageEnView1.MouseInteractLayers := [ mlCreateTextLayers ];
// Allow user to make selections when there are multiple layers (current layer will be highlighted. Selecting a new layer will highlight and allow selection. Layer movement is possible by dragging the edge of the layer)
ImageEnView1.MouseInteractGeneral := [ miSelect, mlMoveLayers ];
// Allow user to make selections when there are multiple layers, including resizing and rotating layers. To move layers, you must drag and click the layer edge
ImageEnView1.MouseInteractGeneral := [ miSelect, mlMoveLayers, mlResizeLayers, mlRotateLayers ];
// Enable our star stamping mode, which adds a star directly to the image wherever the user clicks
procedure Tfmain.btnAddStampClick(Sender: TObject);
const
Stamp_Border_Color = clRed;
Stamp_Border_Width = 2;
Stamp_Fill_Color = clYellow;
Stamp_Shape = iesStar5;
Stamp_Width = 50;
Stamp_Height = 50;
var
cursorBMP: TIEBitmap;
begin
// Set default style of our layer
// Note: Can also use OnNewLayer event to customize further
ImageEnView1.LayerDefaults.Clear();
ImageEnView1.LayerDefaults.Values[ IELP_BorderColor ] := ColorToString( Stamp_Border_Color );
ImageEnView1.LayerDefaults.Values[ IELP_BorderWidth ] := IntToStr( Stamp_Border_Width );
ImageEnView1.LayerDefaults.Values[ IELP_FillColor ] := ColorToString( Stamp_Fill_Color );
// Easier to use DefaultLayerShape... ImageEnView1.LayerDefaults.Values[ IELP_Shape ] := IntToStr( Ord( Stamp_Shape ));
ImageEnView1.LayerDefaults.Values[ IELP_Width ] := IntToStr( Stamp_Width );
ImageEnView1.LayerDefaults.Values[ IELP_Height ] := IntToStr( Stamp_Height );
// Enable stamp mode, and automatic merging of layers
ImageEnView1.LayerOptions := ImageEnView1.LayerOptions + [ loStampMode, loAutoMergeNewLayers ];
IEGlobalSettings().DefaultLayerShape := Stamp_Shape;
ImageEnView1.MouseInteractLayers := [ mlCreateShapeLayers ];
// Show a matching star shaped cursor
cursorBMP := TIEBitmap.Create( Stamp_Width, Stamp_Height );
try
cursorBMP.FillWithShape( Stamp_Shape, Stamp_Width, Stamp_Height, Stamp_Border_Color, Stamp_Border_Width, Stamp_Fill_Color, True, False );
ImageEnView1.SetZoneCursorBitmap( cursorBMP );
finally
cursorBMP.Free;
end;
end;
Compatibility Notes
Prior to v8.6.0, all mouse interactions (both
MouseInteractGeneral and
MouseInteractLayers items) were set with a single property
MouseInteract. To overcome the size limitation for published sets, this was split into:
◼MouseInteractGeneral: All mouse interactions that are
not related to layer editing
◼MouseInteractLayers: Mouse interactions for layer editing
In most cases, code that was previously:
ImageEnView1.MouseInteract := [ miZoom, miScroll ];
Simply changes to:
ImageEnView1.MouseInteractGeneral := [ miZoom, miScroll ];
However for layer editing items (miMoveLayers, miResizeLayers, miRotateLayers, miCreate*Layers, etc.), the prefix has changed to ml, and you must reference
MouseInteractLayers.
In older versions:
ImageEnView1.MouseInteract := [ miMoveLayers, miResizeLayers ];
Now becomes:
ImageEnView1.MouseInteractLayers := [ mlMoveLayers, mlResizeLayers ];
The old layer editing items: miMoveLayers, miResizeLayers, miRotateLayers, miCreateImageLayers, miCreateShapeLayers, miCreateLineLayers, miCreatePolylineLayers, miCreateTextLayers, miClickCreateLineLayers, miClickCreatePolylineLayers, miDrawCreatePolylineLayers, miEditLayerPoints
Now named: mlMoveLayers, mlResizeLayers, mlRotateLayers, mlCreateImageLayers, mlCreateShapeLayers, mlCreateLineLayers, mlCreatePolylineLayers, mlCreateTextLayers, mlClickCreateLineLayers, mlClickCreatePolylineLayers, mlDrawCreatePolylineLayers, mlEditLayerPoints
See Also
◼SelectionOptions
◼LayerDefaults
◼LayersAutoClosePolylines
◼OnUserInteraction
◼OnLayerNotifyEx
◼TIEUserInteraction
◼MouseInteractGeneral