TIECanvas.AdvancedDrawText
Declaration
// Full Overload
function AdvancedDrawText(X, Y, W, H : Integer;
const Text: WideString;
Font: TFont;
TextAngle: Integer = 0;
TextAlign: TIEAlignment = iejLeft;
TextLayout: TIELayout = ielTop;
// Advanced Styling
TextBorderWidth: Integer = 0;
TextBorderColor: TColor = clBlack;
TextFillTransparency1: Integer = 255;
TextFillTransparency2: Integer = 255;
TextFillColor2: TColor = clRed;
TextFillGradient: TIEGDIPlusGradient = gpgNone;
// Background Shape
FrameShape: TIEShape = iesRectangle;
FrameBorderWidth: Integer = 0;
FrameBorderColor: TColor = clBlack;
FrameFillColor: TColor = clNone;
FrameFillColor2: TColor = clRed;
FrameFillGradient: TIEGDIPlusGradient = gpgNone;
FrameMargin: Double = 0.50;
TextVertOffset: Double = 0.0;
// Other parameters
WordWrap: Boolean = False;
AutoShrink: boolean = False;
AntiAlias: Boolean = True;
CalculateOnly: Boolean = False
): TSize; overload;
// Advanced text styling overload
function AdvancedDrawText(X, Y, W, H : Integer;
const Text: WideString;
Font: TFont;
TextAngle: Integer;
TextAlign: TIEAlignment;
TextLayout: TIELayout;
// Advanced Styling
TextBorderWidth: Integer;
TextBorderColor: TColor;
TextFillTransparency1: Integer;
TextFillTransparency2: Integer;
TextFillColor2: TColor;
TextFillGradient: TIEGDIPlusGradient;
// Other parameters
WordWrap: Boolean;
AutoShrink: boolean = False;
AntiAlias: Boolean = True;
CalculateOnly: Boolean = False
): TSize; overload;
// Background shape overload
function AdvancedDrawText(X, Y, W, H : Integer;
const Text: WideString;
Font: TFont;
TextAngle: Integer;
TextAlign: TIEAlignment;
TextLayout: TIELayout;
// Background Shape
FrameShape: TIEShape;
FrameBorderWidth: Integer = 0;
FrameBorderColor: TColor = clBlack;
FrameFillColor: TColor = clNone;
FrameFillColor2: TColor = clRed;
FrameFillGradient: TIEGDIPlusGradient = gpgNone;
FrameMargin: Double = 0.50;
TextVertOffset: Double = 0.0;
// Other parameters
WordWrap: Boolean = False;
AutoShrink: boolean = False;
AntiAlias: Boolean = True;
CalculateOnly: Boolean = False
): TSize; overload;
Description
Draw text at a specified position with advanced options, including:
◼Outlined text (text has a border and optional fill)
◼Background frame of many shapes and gradients
◼Auto-shrinking of wide text
For a simpler method, use:
DrawText.
Text Properties
Parameter | Description |
X | Horizontal position |
Y | Vertical position |
W | Optional: Specify 0, or the maximum width for the text to enforce text wrapping and auto-shrinking |
H | Optional: Specify 0, or the maximum height for the text to enforce auto-shrinking |
Text | The string to output |
Font | Optional: The font for the text. You can create a temporary TFont object, or specify NIL to use the Canvas.Font |
TextAngle | The rotation of the text in in degrees counter-clockwise, e.g. -45 will draws text diagonally downwards |
TextAlign | The horizontal alignment of the text within the rect of the specified width. Specify iejLeft to position at X * |
TextLayout | The vertical alignment of the text within the rect of the specified height. Specify ielTop to position at Y * |
Advanced Text Styling
Parameter | Description |
TextBorderWidth | If not 0 the text will have a colored border of the specified width |
TextBorderColor | The color of the text border |
TextFillTransparency1 | The transparency of the text fill (the color is specified by Font.Color) |
TextFillTransparency2 | The transparency of the secondary fill (if the text fill has a gradient) |
TextFillColor2 | The color of the secondary fill (if the text fill has a gradient). The fill color is specified by Font.Color. gpgNone specifies no gradient |
TextFillGradient | The direction of the gradient for the text fill (from Font.Color to TextFillColor2) |
Text Background Frame
Parameter | Description |
FrameShape | The shape of frame |
FrameBorderWidth | If not 0 then there will be a shape drawn behind the text of the specified width |
FrameBorderColor | The color of the frame border (or clNone for no border) |
FrameFillColor | The color of the frame fill (or clNone for no fill) |
FrameFillColor2 | The color of the secondary fill (if the frame fill has a gradient) |
FrameFillGradient | The direction of the gradient for the frame fill (from FrameFillColor to FrameFillColor2). gpgNone specifies no gradient |
FrameMargin | Specifies the amount to increase the size of the shape (if width and height are auto-calculated) so the border does not overlap the text. This value is a percentage, e.g. 0.50 adds margins of 50% of the text width and height |
TextVertOffset | Shifts the drawing of text up or down. This is a percentage value with positive and negative values, so -0.10, moves the text up 10% of the layer height. 0.50 will shift halfway down. direction of the gradient for the frame fill (from FrameFillColor to FrameFillColor2). gpgNone specifies no gradient |
Other Parameters
Parameter | Description |
WordWrap | If true, and the text is wider than the W value, it will wrap onto the next line * |
AutoShrink | If True, and the text is larger than the rect specified by W x H, the font size will be reduced until it fits * |
AntiAlias | True uses best quality font. False disables anti-aliasing |
CalculateOnly | If true, the text is not drawn, just the output size returned as the result |
* These parameters have no effect unless the W and H values have been specifically set (i.e. are not 0)
Result is the size of the draw area, i.e. if you have specified a frame, then the result is the size of the frame in pixels. Otherwise the result is the size of the text in pixels.
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
ImageEnView1.IEBitmap.IECanvas.Font.Name := 'Tahoma';
ImageEnView1.IEBitmap.IECanvas.Font.Size := 14;
ImageEnView1.IEBitmap.IECanvas.Font.Style := [fsBold];
ImageEnView1.IEBitmap.IECanvas.AdvancedDrawText( 100, 100, 250, 100,
'EXPLOSION!', nil,
0, iejCenter, ielCenter,
iesExplosion, 1, clBlack,
clRed, clYellow, gpgVertCenter );
ImageEnView1.Update();
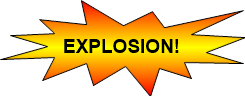
ImageEnView1.IEBitmap.IECanvas.Font.Name := 'Tahoma';
ImageEnView1.IEBitmap.IECanvas.Font.Size := 22;
ImageEnView1.IEBitmap.IECanvas.Font.Style := [fsBold];
ImageEnView1.IEBitmap.IECanvas.AdvancedDrawText( 100, 100, 230, 200,
'I Heart Delphi', nil,
0, iejCenter, ielCenter,
iesHeart, 0, clNone, $004646FF,
clRed, gpgNone, -0.15 );
ImageEnView1.Update();
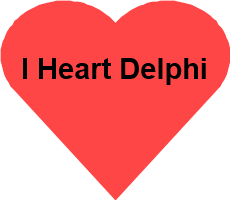
// Yellow text with red border
ImageEnView1.IEBitmap.IECanvas.Font.Name := 'Tahoma';
ImageEnView1.IEBitmap.IECanvas.Font.Size := 54;
ImageEnView1.IEBitmap.IECanvas.Font.Style := [fsBold];
ImageEnView1.IEBitmap.IECanvas.Font.Color := clYellow;
ImageEnView1.IEBitmap.IECanvas.AdvancedDrawText( 100, 100, 0, 0,
'ImageEn!', nil,
0, iejLeft, ielTop,
3, clRed, 255, 255, clBlack, gpgNone );
ImageEnView1.Update();
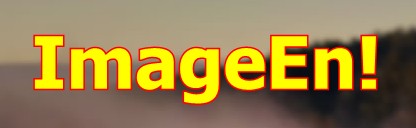
// Semi-transparent white text with red border
ImageEnView1.IEBitmap.IECanvas.Font.Name := 'Tahoma';
ImageEnView1.IEBitmap.IECanvas.Font.Size := 54;
ImageEnView1.IEBitmap.IECanvas.Font.Style := [fsBold];
ImageEnView1.IEBitmap.IECanvas.Font.Color := clYellow;
ImageEnView1.IEBitmap.IECanvas.AdvancedDrawText( 100, 100, 0, 0,
'ImageEn!', nil,
0, iejLeft, ielTop,
3, clRed,
IEOpacityToAlphaD( 0.3 ), // 30% opaque
255, clBlack, gpgNone );
ImageEnView1.Update();
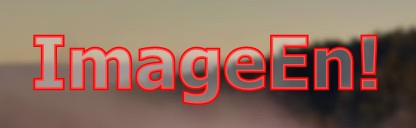
// Transparent text with yellow border
ImageEnView1.IEBitmap.IECanvas.Font.Name := 'Tahoma';
ImageEnView1.IEBitmap.IECanvas.Font.Size := 54;
ImageEnView1.IEBitmap.IECanvas.Font.Style := [fsBold];
ImageEnView1.IEBitmap.IECanvas.Font.Color := clYellow;
ImageEnView1.IEBitmap.IECanvas.AdvancedDrawText( 100, 100, 0, 0,
'ImageEn!', nil,
0, iejLeft, ielTop,
3, clYellow,
0, // 100% transparent
255, clBlack, gpgNone );
ImageEnView1.Update();
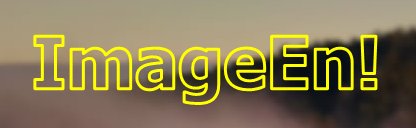
// Gradient text with border
ImageEnView1.IEBitmap.IECanvas.Font.Name := 'Tahoma';
ImageEnView1.IEBitmap.IECanvas.Font.Size := 54;
ImageEnView1.IEBitmap.IECanvas.Font.Style := [fsBold];
ImageEnView1.IEBitmap.IECanvas.Font.Color := clRed;
ImageEnView1.IEBitmap.IECanvas.AdvancedDrawText( 100, 100, 0, 0,
'ImageEn!', nil,
0, iejLeft, ielTop,
3, clBlack,
255, 255, clYellow, gpgVertical );
ImageEnView1.Update();
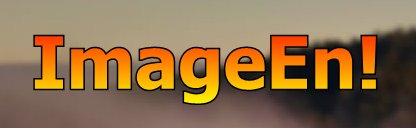
// Centered gradient text without border
ImageEnView1.IEBitmap.IECanvas.Font.Name := 'Tahoma';
ImageEnView1.IEBitmap.IECanvas.Font.Size := 54;
ImageEnView1.IEBitmap.IECanvas.Font.Style := [fsBold];
ImageEnView1.IEBitmap.IECanvas.Font.Color := clRed;
ImageEnView1.IEBitmap.IECanvas.AdvancedDrawText( 100, 100, 0, 0,
'ImageEn!', nil,
0, iejLeft, ielTop,
0, clBlack,
255, 255, clWhite, gpgVertCenter );
ImageEnView1.Update();
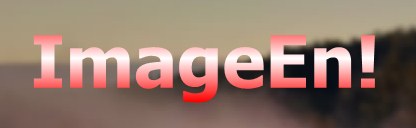
// Text with gradient ranging from 0% to 70% opacity
ImageEnView1.IEBitmap.IECanvas.Font.Name := 'Tahoma';
ImageEnView1.IEBitmap.IECanvas.Font.Size := 54;
ImageEnView1.IEBitmap.IECanvas.Font.Style := [fsBold];
ImageEnView1.IEBitmap.IECanvas.AdvancedDrawText( 100, 100, 0, 0,
'ImageEn!', nil,
0, iejLeft, ielTop,
0, clBlack,
0, // 100% transparent
IEOpacityToAlphaD( 0.7 ), // 70% opacity
clYellow, gpgHorizontal );
ImageEnView1.Update();
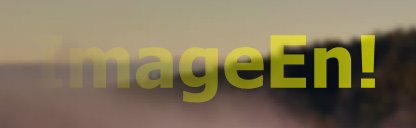
// Draw text with background to image
ImageEnView1.IEBitmap.IECanvas.Font.Name := 'Tahoma';
ImageEnView1.IEBitmap.IECanvas.Font.Height := 50;
ImageEnView1.IEBitmap.IECanvas.Font.Style := [fsBold];
ImageEnView1.IEBitmap.IECanvas.Font.Color := Color;
ImageEnView1.IEBitmap.IECanvas.AdvancedDrawText( 200, 20, 90, 150,
'I do!', // Text
NIL, // Use canvas font
0, iejCenter, ielCenter, // Angle and alignment
iesSpeechBubbleLeftOutShort, // Background Shape
2, clBlack, // FrameBorderWidth/FrameBorderColor
clWhite, clWhite, gpgNone, // FrameFillColor/FrameFillColor2/FrameFillGradient
0.33, // Reduced margin
-0.2, // Move text up
False, // WordWrap
True ); // AutoShrink
ImageEnView1.Update(); // Show changes in our viewer
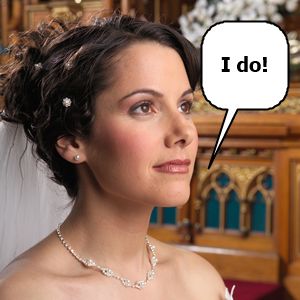
See Also
◼DrawText
◼AdvancedDrawAngle
◼AdvancedDrawLine
◼AdvancedDrawPolyline
◼AdvancedDrawShape