Declaration
procedure Rectangle(X1, Y1, X2, Y2: Integer); overload;
procedure Rectangle(const Rect: TRect); overload;
Description
Draw a rectangle within a specified area.
The rectangle border is set by
Pen and the fill by
Brush.
GDI+ Methods: GdipFillRectangle, GdipDrawRectangle
Note: The X2 and Y2 points are excluded from the painting
// Draw an envelope with 50% transparency
with ImageEnView1.IEBitmap.IECanvas do
begin
Pen.Mode := pmCopy;
Pen.Style := psSolid;
Pen.Color := clBlack;
Pen.Transparency := 128;
Brush.Color := clYellow;
Brush.Style := bsSolid;
Brush.Transparency := 128;
// Draw outer rect
Rectangle( 100, 100, 500, 300 );
// Draw flap
MoveTo( 100, 100 );
LineTo( 300, 200 );
LineTo( 500, 100 );
end;
ImageEnView1.Update();
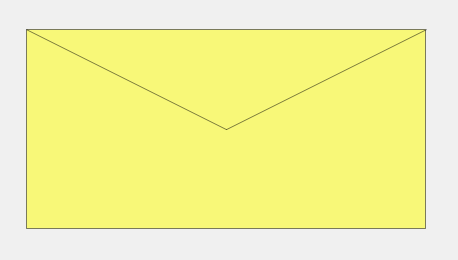
// Draw a semi-transparent text box onto a bitmap
const
Horz_Margin = 8;
Vert_Margin = 3;
Center_Text = False;
var
x, y: integer;
tw, rw, rh: integer;
iec: TIECanvas;
ss: string;
begin
ss := 'This is my text';
x := 100;
y := 100;
iec := TIECanvas.Create( Bitmap.Canvas );
iec.Font.Size := 30;
iec.Font.Style := [fsBold];
tw := iec.TextWidth(ss);
rw := imax( tw + 2 * Horz_Margin, iec.TextWidth( ss ) + 2 * Horz_Margin );
rh := iec.TextHeight(ss) + 2 * Vert_Margin;
if Center_Text then
dec( x, rw div 2 );
iec.Brush.Color := clYellow;
iec.Brush.Style := bsSolid;
iec.Brush.Transparency := 196;
iec.Pen.Color := clBlack;
iec.Pen.Style := psSolid;
iec.Rectangle( x, y, x + rw, y + rh );
iec.Brush.Style := bsClear;
iec.TextOut(x + ( rw - tw ) div 2, y + Vert_Margin, ss);
iec.Free;
end;
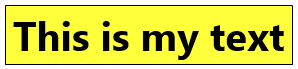
See Also
◼AdvancedDrawShape