TIEVisionOCR.getOrientation
Declaration
procedure getOrientation(out orientation: TIEVisionOCROrientation; out writingDirection: TIEVisionOCRWritingDirection; out textlineOrder: TIEVisionOCRTextlineOrder; out deskewAngle: single); safecall;
Description
Returns detail of the orientation, rotation and text direction of the document. This can be used for non-OCR applications, such as automatically correcting the rotation of scanned documents.
getOrientation must be called after
getTextAngle or
recognize.
Parameter | Description |
orientation | Character orientation, mainly used for document orientation, e.g. a page scanned upside-down would return ievOCROrientPAGE_DOWN |
writingDirection | Writing direction |
textlineOrder | Line direction, e.g. Right-to-left for Arabic or Hebrew, or Top-to-bottom for some Asian text |
deskewAngle | Deskew angle in radians in the range -Pi/4 and Pi/4. The level of rotation required to correct a document scanned at an angle |
Note:
◼ getOrientation works best with the
ievOCRAuto_OSD segmentation mode
◼ getOrientation requires the support file
Osd.TrainedData for all languages
Example
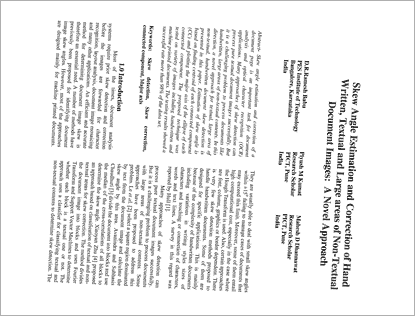
// AUTOMATICALLY ROTATE CURRENT DOCUMENT IMAGE TO CORRECT ORIENTATION
// Create OCR Engine
m_OCR := IEVisionLib.createOCR( sOcrFilesPath, IEOCRLanguageList[ OCR_English_language ].Code, ievOCRDefault );
// Detect orientation
m_OCR.setSegmentationMode( ievOCRAuto_OSD );
m_OCR.getTextAngle( ImageEnView1.IEBitmap.GetIEVisionImage() ); // Must call either getTextAngle() or recognize() before GetOrientation()
// Retrieve orientation details
m_OCR.getOrientation( orientation, writingDirection, textlineOrder, deskewAngle );
{
Results for above image:
orientation : ievOCROrientPAGE_RIGHT
writingDirection : ievOCRWritDirLEFT_TO_RIGHT
textlineOrder : ievOCRTxtlineOrderTOP_TO_BOTTOM
deskewAngle : -2.94 (which is -1.5 deg.)
}
// Calculate rotation angle
rotAngle := -deskewAngle * 180 / PI;
if orientation = ievOCROrientPAGE_RIGHT then
rotAngle := rotAngle - 90
else
if orientation = ievOCROrientPAGE_DOWN then
rotAngle := rotAngle - 180
else
if orientation = ievOCROrientPAGE_LEFT then
rotAngle := rotAngle - 270;
{
Result for above image:
rotAngle : -92.94 deg.
}
// Now rotate the image
if rotAngle <> 0 then
ImageEnView1.Proc.Rotate( -rotAngle );