TIEBitmap.RenderToTIEBitmapEx
Declaration
procedure RenderToTIEBitmapEx(Dest: TIEBitmap; xDst, yDst, dxDst, dyDst: integer; xSrc, ySrc, dxSrc, dySrc: integer; bEnableAlpha: Boolean = True; Transparency: integer = 255; Filter: TResampleFilter = rfNone; RenderOperation: TIERenderOperation = ielNormal; Opacity: double = 1.0);
Description
Draws the rectangle from xSrc, ySrc and size dxSrc, dySrc inside the TIEBitmap destination rectangle at xDst, yDst and size: dxDst, dyDst of
Dest.
The
PixelFormat of
Dest must be ie24RGB. The source image can be any
PixelFormat.
If
bEnableAlpha is true, then rendering will support the
AlphaChannel, i.e. painting will not occur for areas of Alpha < 255.
Transparency specifies the transparency value (0=Fully Transparent to 255=Fully Opaque).
Filter the resampling filter.
RenderOperation the rendering operation.
Opacity specifies the opacity (0=Fully Transparent to 1.0=Fully Opaque).
Note: RenderToTIEBitmapEx and
DrawToTIEBitmap are simplified versions of
RenderToTIEBitmap
Opacity vs Transparency
Both the Opacity and Transparency parameters provide the same functionality. Transparency is the traditional ImageEn value, whereas Opacity provides easier PSD compatibility.
While they can be used in combination, generally only one will be used, i.e. leave Opacity=1 and make use of transparency, or alternatively, leave Transparency=255 and make use of Opacity. For example, for 50% opacity: Transparency = 255 and Opacity = 0.5, or Transparency = 128 and Opacity = 1.0
// Render an image in a TImageEnView to a TIEBitmap at half-size
bmp.Allocate( ImageEnView1.IEBitmap.Width div 2, ImageEnView1.IEBitmap.Height div 2 );
ImageEnView1.IEBitmap.RenderToTIEBitmapEx( bmp,
0, 0, bmp.Width, bmp.Height,
0, 0, ImageEnView1.IEBitmap.Width, ImageEnView1.IEBitmap.Height,
True, 255, rfLanczos3 );
// Merge images of ImageEnView1 and ImageEnView2 using "InverseColorDodge" and put onto ImageEnView3
ImageEnView3.IEBitmap.Assign( ImageEnView2.IEBitmap );
ImageEnView1.IEBitmap.RenderToTIEBitmapEx( ImageEnView3.IEBitmap,
0, 0, ImageEnView2.IEBitmap.Width, ImageEnView2.IEBitmap.Height,
0, 0, ImageEnView1.IEBitmap.Width, ImageEnView1.IEBitmap.Height,
False, 255, rfNone,
ielInverseColorDodge );
ImageEnView3.Update();
// Add watermark in bottom left corner of image at 30% opacity
bmpImg := TIEBitmap.Create();
bmpWM := TIEBitmap.Create();
bmpImg.LoadFromFile('D:\image.jpeg');
bmpWM.LoadFromFile('D:\Watermark.png');
wmOpacity := 0.30;
bmpWM.RenderToTIEBitmapEx( bmpImg,
0, bmpImg.Height - bmpWM.Height, bmpWM.Width, bmpWM.Height,
0, 0, bmpWM.Width, bmpWM.Height,
True, 255, rfFastLinear, ielNormal,
wmOpacity );
bmpImg.SaveToFile('D:\image-marked.jpeg');
bmpImg.Free;
bmWM.Free;
// Overlay entire image with watermark at 30% opacity (maintaining aspect ratio)
bmpImg := TIEBitmap.Create();
bmpWM := TIEBitmap.Create();
bmpImg.LoadFromFile('D:\image.jpeg');
bmpWM.LoadFromFile('D:\Watermark.png');
aRect := GetImageRectWithinArea( wmBMP.Width, wmBMP.Height, bmpImg.Width, bmpImg.Height);
wmOpacity := 0.30;
bmpWM.RenderToTIEBitmapEx( bmpImg,
aRect.Left, aRect.Top, aRect.Right - aRect.Left, aRect.Bottom - aRect.Top,
0, 0, bmpWM.Width, bmpWM.Height,
True, 255, rfFastLinear, ielNormal,
wmOpacity );
bmpImg.SaveToFile('D:\image-marked.jpeg');
bmpImg.Free;
bmWM.Free;
// CREATE BITMAP WITH BLURRED IMAGE FILLING NON-IMAGE AREA
// DestBmp: Output image for result
// BackgroundBmp: Image to use as background (must be valid)
// ImageBmp: Image to draw centered (can be same as BackgroundBmp, or can be NIL)
// Width, Height: Size to make DestBmp
// BlurRadius: How much to blur image (Default is 8, 0 for no blur)
// BackgroundOpacity: If less than 1.0, the background is drawn semi-opaque (with BackgroundColor visible)
procedure StretchDrawWithBlur(DestBmp, BackgroundBmp, ImageBmp: TIEBitmap; Width, Height: Integer; BlurRadius: Integer = 8; BackgroundOpacity: Double = 0.75; BackgroundColor: TColor = clBlack);
var
aRect: TRect;
DestRect : TIERectangle;
begin
// Allocate and size
if not assigned( DestBmp ) then
DestBmp := TIEBitmap.Create();
DestBmp.Allocate( Width, Height );
// Fill background color if needed
if BackgroundOpacity < 1.0 then
DestBmp.Fill( BackgroundColor );
// Scale draw the background to fill entire image area
aRect := GetImageRectWithinArea( BackgroundBmp.Width, BackgroundBmp.Height, DestBmp.Width, DestBmp.Height,
0, 0, True, True, True, True, 0,
_fmFillRect_WithOverlap );
DestRect := IERectangle( aRect );
BackgroundBmp.RenderToTIEBitmapEx( DestBmp, DestRect.X, DestRect.Y, DestRect.Width, DestRect.Height,
0, 0, BackgroundBmp.Width, BackgroundBmp.Height,
True, 255, rfNone, ielNormal, BackgroundOpacity );
// Blur the background
if BlurRadius > 0 then
with TImageEnProc.CreateFromBitmap( DestBmp ) do
begin
Blur( BlurRadius );
Free();
end;
// Draw our image
if ImageBmp <> nil then
begin
aRect := GetImageRectWithinArea( ImageBmp.Width, ImageBmp.Height, DestBmp.Width, DestBmp.Height );
DestRect := IERectangle( aRect );
ImageBmp.RenderToTIEBitmapEx( DestBmp, DestRect.X, DestRect.Y, DestRect.Width, DestRect.Height,
0, 0, ImageBmp.Width, ImageBmp.Height,
True, 255, rfNone, ielNormal, 1.0 );
end;
end;
// TEST METHOD
procedure TImageEnViewForm.Button1Click(Sender: TObject);
var
bmpIn, bmpOut: TIEBitmap;
begin
bmpIn := TIEBitmap.Create();
bmpOut := TIEBitmap.Create();
try
bmpIn.LoadFromFile( 'D:\image.jpeg' );
StretchDrawWithBlur( bmpOut, bmpIn, bmpIn, 900, 400, 8, 0.75, clGray );
ImageEnView1.Assign( bmpOut );
finally
bmpIn.Free;
bmpOut.Free;
end;
end;
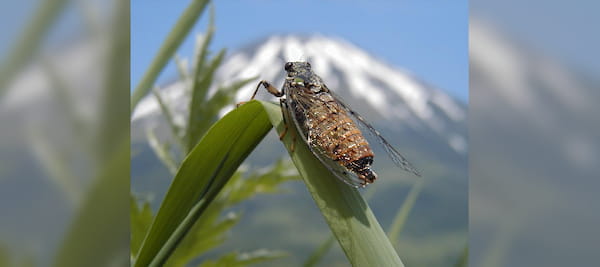
TIEBitmap Assignment and Drawing Methods
TIEBitmap Methods
Method | Mode | Purpose |
Assign | From TIEBitmap/TBitmap/TGraphic/TIcon | Copy whole image |
AssignImage | From TIEBitmap | Like assign, but does not copy the alpha channel |
AssignRect | From TIEBitmap/TBitmap | Copy a specified rect |
CopyAndConvertFormat | From TIEBitmap | Copy whole image |
CopyRectTo | To TIEBitmap | Copy rect to another image (without scaling) |
CopyWithMask1 | To TIEBitmap | Copy image using a mask to specify what is copied from the source |
CopyWithMask2 | To TIEBitmap | Copy image using a mask to specify what is replaced in the destintation |
DrawToTIEBitmap | To TIEBitmap | Copies all or part of the image to a specified position and/or size |
JoinBitmaps | From two TIEBitmaps | Draws two bitmaps to a single bitmap |
MergeAlphaRectTo | With TIEBitmap | Merges the alpha channels of two TIEBitmaps using merge rules |
MergeWithAlpha | With TIEBitmap | Merges all or part of two TIEBitmaps with alpha channels to a specified position |
RenderToTIEBitmapEx | To TIEBitmap | Extended drawing of content to a TIEBitmap |
StretchRectTo | To TIEBitmap | Copy rect to dest rect in another image (with scaling) |
SwitchTo | To TIEBitmap | Move content from one TIEBitmap to another |
TBitmap Methods
Method | Mode | Purpose |
Assign | From TIEBitmap/TBitmap/TGraphic/TIcon | Copy whole image |
AssignTo | To TIEBitmap/TBitmap | Copy whole image with optional scaling |
AssignRect | From TIEBitmap/TBitmap | Copy a specified rect |
CopyFromTBitmap | From TBitmap | Copy whole image |
CopyToTBitmap | To TBitmap | Copy whole image |
RenderToTBitmapEx | To TBitmap | Extended drawing of content to a TBitmap |
TCanvas Methods
Compatibility Information
In v6.0.0 the
bEnableAlpha parameter was added. Set this to true to maintain existing functionality.
See Also
◼RenderToTIEBitmap
◼DrawToTIEBitmap