ImageEn, unit iexUserInteractions |
|
TIERetouchToolInteraction
Declaration
TIERetouchToolInteraction = class(TIECursorToolInteraction);
Description
A class of
TIEUserInteraction that controls interaction for the Retouch tool (when
MouseInteractGeneral is
miRetouchTool).
The retouch tool allows the user to apply fix effects to the image, e.g. smudging, blurring, inpainting, etc. When used with a tablet/pen, then
pressure may affect the brush size (except for the smudge and motion blur effects).
Retouch Modes
The following
tools are available:
Effect | Description | Properties | More Info |
iermSmudge | Smudge brushed area | SmudgePressure, SmudgeBlur | - |
iermBlur | Blur brushed area | BlurRadius | Blur |
iermMotionBlur | Apply a motion blur effect | MotionBlurAngle, MotionBlurRadius, MotionBlurSigma | MotionBlur |
iermAutoSharpen | Sharpen contours of brushed area | AutoSharpenIntensity, AutoSharpenRate | AutoSharp |
iermSharpen | Apply sharpening filter to brushed area | SharpenIntensity, SharpenNeighbourhood | Sharpen |
iermUnsharpen | Apply a gaussian blur to brushed area | UnsharpenAmount, UnsharpenRadius, UnsharpenThreshold | UnsharpMask |
iermSmooth | Apply SNN noise reduction to brushed area | SmoothRadius | SymmetricNearestNeighbour |
iermPixelize | Convert brushed area to large pixel blocks | PixelizeBlockSize | Pixelize |
iermBrightness | Adjust brightness of brushed area | BrightnessAdjustment | IntensityRGBAll |
iermSaturation | Adjust color saturation of brushed area | SaturationAmount | AdjustSaturation |
iermWave | Apply a wave effect to brushed area | WaveAmplitude, WaveLength, WavePhase | Wave |
iermPencilSketch | Make brushed area resemble a pencil sketch (Note 1) | PencilSketchAlpha, PencilSketchDensity, PencilSketchGray, PencilSketchLevels, PencilSketchThickness | PencilSketch |
iermIEVisionInpaint | Restore brushed area using the outer region (fill missing areas of image or erase blemishes) (Note 2) | InpaintMethod, InpaintRangeSize | inpaint |
iermIEVisionSmooth | Apply NLM noise reduction to brushed area (Note 3) | DenoiseFilterStrength | fastNlMeansDenoisingColored |
iermWarp | Warp the image by pushing the area under the cursor | - | Warp |
iermSwirl | Apply a rotational effect under the cursor to obfuscate the image | - | SwirlTwists |
iermCustom | Apply a custom effect to brushed area | | OnCustomRetouch |
Note:
1. iermPencilSketch and iermSwirl can be very slow and require a lot of memory
2. iermIEVisionInpaint requires
IEVision. If IEVision is not available, the iermSmudge method is used instead
3. iermIEVisionSmooth requires
IEVision. If IEVision is not available, the iermSmooth method is used instead
4. iermSwirl is always circular (1:1) so may draw outside the retouched area
| Demos\ImageEditing\RetouchTool\RetouchTool.dpr |
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
| Demos\ImageEditing\WarpBrush\WarpBrush.dpr |
General Properties
Smudge Tool Properties
Blur Tool Properties
Motion Blur Tool Properties
Auto Sharpen Tool Properties
Sharpen Tool Properties
Unsharpen Tool Properties
Smooth Tool Properties
Pixelize Tool Properties
Brightness Tool Properties
Saturation Tool Properties
Wave Tool Properties
Swirl Tool Properties
Pencil Sketch Tool Properties
Inpaint Tool Properties
Denoise Tool Properties
Retouching Operation
Other
// Enable retouch mode
ImageEnView1.MouseInteractGeneral := [miRetouchTool];
// Smudge the image
ImageEnView1.RetouchTool.RetouchMode := iermSmudge;
ImageEnView1.RetouchTool.SmudgePressure := 15;
ImageEnView1.RetouchTool.Feathering := 3;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
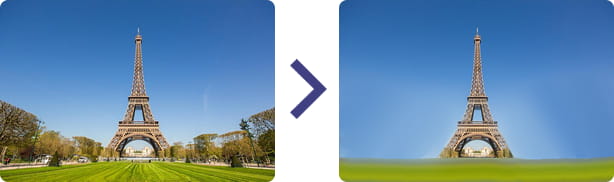
// Apply motion blur to image
ImageEnView1.RetouchTool.RetouchMode := iermMotionBlur ;
ImageEnView1.RetouchTool.MotionBlurSigma := 8;
ImageEnView1.RetouchTool.MotionBlurAngle := 180;
ImageEnView1.RetouchTool.MotionBlurRadius := 10;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
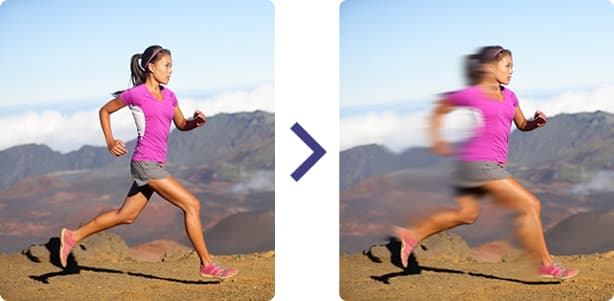
// Use IEVision's Inpainting to remove blemishes in the image
ImageEnView1.RetouchTool.RetouchMode := iermIEVisionInpaint;
ImageEnView1.RetouchTool.InpaintRangeSize := 6;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
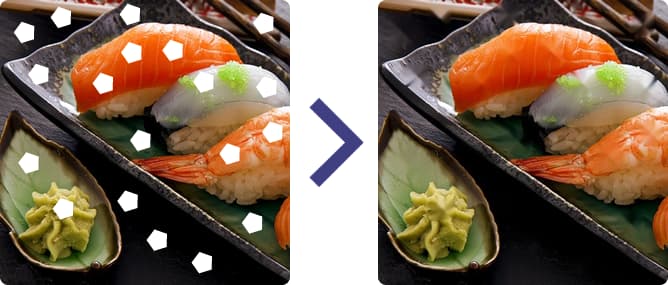
// Pixelate the brushed area (e.g. to hide sensitive detail)
ImageEnView1.RetouchTool.RetouchMode := iermPixelize;
ImageEnView1.RetouchTool.PixelizeBlockSize := 5;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
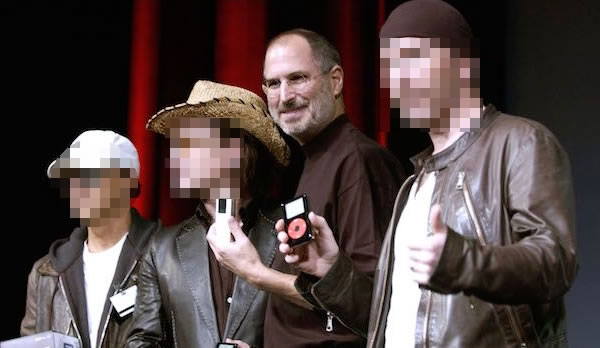
// Warp the image
ImageEnView1.RetouchTool.RetouchMode := iermWarp;
ImageEnView1.RetouchTool.BrushSize := 60;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
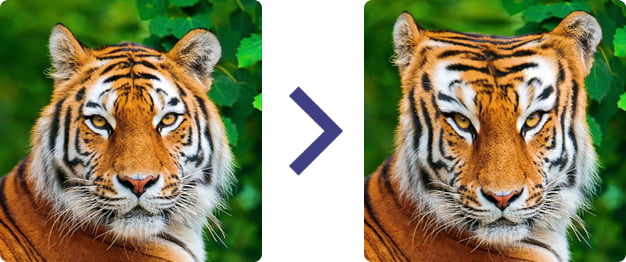
// Blur the image
ImageEnView1.RetouchTool.RetouchMode := iermBlur;
ImageEnView1.RetouchTool.BlurRadius := 7;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
// Sharpen the image
ImageEnView1.RetouchTool.RetouchMode := iermAutoSharpen;
ImageEnView1.RetouchTool.AutoSharpenRate := 0.025;
ImageEnView1.RetouchTool.AutoSharpenIntensity := 30;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
// Remove noise from the image
ImageEnView1.RetouchTool.RetouchMode := iermSmooth;
ImageEnView1.RetouchTool.SmoothRadius := 12;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
// Lighten the brushed region
ImageEnView1.RetouchTool.RetouchMode := iermBrightness;
ImageEnView1.RetouchTool.BrightnessAdjustment := -10;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
// Reduce color saturation in the brushed image
ImageEnView1.RetouchTool.RetouchMode := iermSaturation;
ImageEnView1.RetouchTool.SaturationAmount := -30;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
// Apply a wave effect to the brushed region
ImageEnView1.RetouchTool.RetouchMode := iermWave;
ImageEnView1.RetouchTool.WaveAmplitude := 5;
ImageEnView1.RetouchTool.WavePhase := 90;
ImageEnView1.RetouchTool.WaveLength := 20;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
// Apply a gray-scale pencil sketch effect to brushed areas
ImageEnView1.RetouchTool.RetouchMode := iermPencilSketch;
ImageEnView1.RetouchTool.PencilSketchGray := True;
ImageEnView1.RetouchTool.PencilSketchLevels := 3;
ImageEnView1.RetouchTool.PencilSketchThickness := 1.4;
ImageEnView1.RetouchTool.PencilSketchAlpha := 0.2;
ImageEnView1.RetouchTool.PencilSketchDensity := 0.8;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
// Show retouch brush properties dialog
ImageEnView1.MouseInteractGeneral := [miRetouchTool];
ImageEnView1.BrushShowPropertiesDialog();
See Also
◼ImageEn Interactive Tools
◼BrushShowPropertiesDialog
◼OnUserInteraction
◼TImageEnView Actions